PHP Array Exercises : Check whether all array values are strings or not
PHP Array: Exercise-46 with Solution
Write a PHP function to check whether all array values are strings or not.
Sample Solution:
PHP Code:
<?php
// Function to check if all elements in an array are strings
function check_strings_in_array($arr)
{
// Use array_map to check if each element is a string, then sum the results
// If the sum is equal to the total count of elements, it means all elements are strings
return array_sum(array_map('is_string', $arr)) == count($arr);
}
// Test arrays
$arr1 = array('PHP', 'JS', 'Python');
$arr2 = array('SQL', 200, 'MySQL');
// Check and display the result for $arr1
var_dump(check_strings_in_array($arr1));
// Check and display the result for $arr2
var_dump(check_strings_in_array($arr2));
?>
Output:
bool(true) bool(false)
Flowchart:
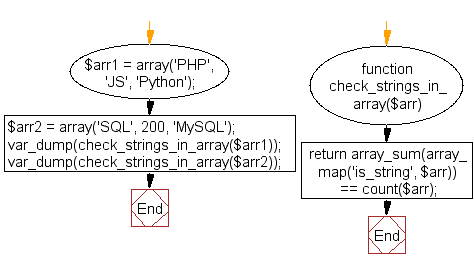
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to do a multi-dimensional difference, i.e. returns values of the first array that are not in the second array.
Next: Write a PHP function to get an array with the first key and value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics