PHP Array Exercises : Returns values of the first array that are not in the second array
Write a PHP script to do a multi-dimensional difference, i.e. returns values of the first array that are not in the second array.
Sample Solution:
PHP Code:
<?php
// Create two multidimensional arrays with color and corresponding values
$color1 = array( array('Red', 80), array('Green', 70), array('white', 60) );
$color2 = array( array('Green', 70), array('Black', 95) );
// Use array_udiff to find the difference between $color1 and $color2 based on custom comparison
$color = array_udiff($color1, $color2, create_function(
'$a,$b', 'return strcmp( implode("", $a), implode("", $b) ); ')
);
// Print the resulting array after the difference operation
print_r($color);
?>
Output:
Array ( [0] => Array ( [0] => Red [1] => 80 ) [2] => Array ( [0] => white [1] => 60 ) )
Flowchart:
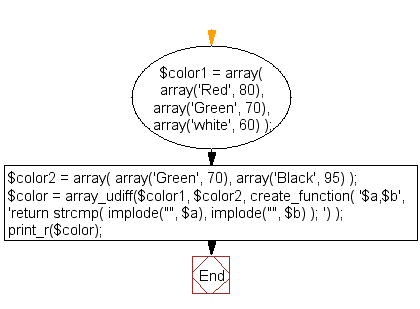
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP a function to remove a specified duplicate entry from an array.
Next: Write a PHP function to check whether all array values are strings or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.