PHP Array Exercises : Remove duplicate values from an array which contains only integers or strings
PHP Array: Exercise-39 with Solution
Write a PHP program to remove duplicate values from an array which contains only strings or only integers.
Sample Solution:
PHP Code:
<?php
// Define an array of colors
$colors = array(
0 => 'Red',
1 => 'Green',
2 => 'White',
3 => 'Black',
4 => 'Red',
);
// Define an array of numbers
$numbers = array(
0 => 100,
1 => 200,
2 => 100,
3 => -10,
4 => -10,
5 => 0,
);
// Use array_flip to swap keys and values, then use array_keys to extract unique values
$uniq_colors = array_keys(array_flip($colors));
// Use array_flip to swap keys and values, then use array_keys to extract unique values
$uniq_numbers = array_keys(array_flip($numbers));
// Display the array of unique colors
print_r($uniq_colors);
// Display the array of unique numbers
print_r($uniq_numbers);
?>
Output:
Array ( [0] => Red [1] => Green [2] => White [3] => Black ) Array ( [0] => 100 [1] => 200 [2] => -10 [3] => 0 )
Flowchart:
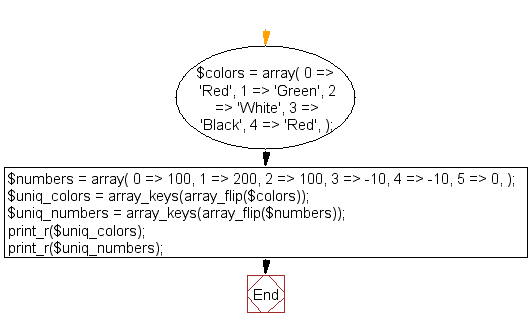
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP function to create a multidimensional unique array for any single key index.
Next: Write a PHP program to get a sorted array without preserving the keys.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics