PHP Array Exercises : Count the total number of times a specific value appears in an array
Write a PHP script to count the total number of times a specific value appears in an array.
Sample Solution:
PHP Code:
<?php
// Define a function to count occurrences of a value in an array
function count_array_values($my_array, $match)
{
$count = 0;
// Iterate through each element in the array
foreach ($my_array as $key => $value)
{
// Check if the current element matches the specified value
if ($value == $match)
{
// Increment the count if a match is found
$count++;
}
}
// Return the total count of occurrences
return $count;
}
// Define an associative array with color values
$colors = array("c1"=>"Red", "c2"=>"Green", "c3"=>"Yellow", "c4"=>"Red");
// Display the count of occurrences of the value "Red" in the array
echo "\n"."Red color appears ".count_array_values($colors, "Red"). " time(s)."."\n";
?>
Output:
Red color appears 2 time(s).
Flowchart:
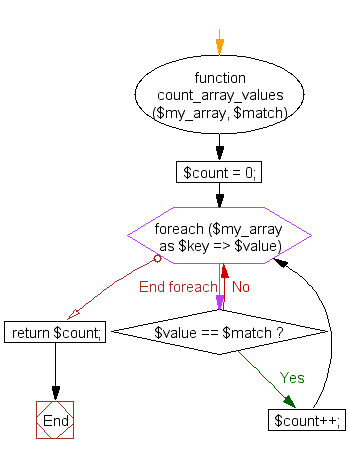
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to lower-case and upper-case, all elements in an array.
Next: Write a PHP function to create a multidimensional unique array for any single key index.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.