PHP Array Exercises : Trim all the elements in an array using array_walk function
PHP Array: Exercise-35 with Solution
Write a PHP script to trim all the elements in an array using array_walk function.
Sample Solution:
PHP Code:
<?php
// Define an array with string values containing extra whitespaces
$colors = array( "Red ", " Green", "Black ", " White ");
// Display the original array using print_r
print_r($colors);
// Use array_walk and create_function to trim whitespaces from each array element
array_walk($colors, create_function('&$val', '$val = trim($val);'));
// Display the modified array after trimming whitespaces
print_r($colors);
?>
Output:
Array ( [0] => Red [1] => Green [2] => Black [3] => White ) Array ( [0] => Red [1] => Green [2] => Black [3] => White )
Flowchart:
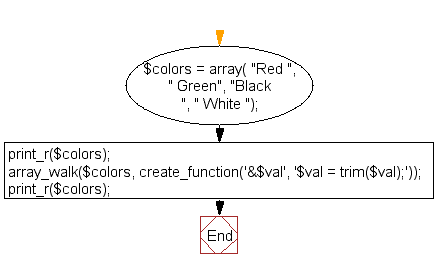
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to sort an associative array (alphanumeric with case-sensitive data) by values.
Next: Write a PHP script to lower-case and upper-case, all elements in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics