PHP Array Exercises : Search a specified value within the values of an associative array
Write a PHP function to search a specified value within the values of an associative array.
Sample Solution:
PHP Code:
<?php
// Define a function named arraysearch that searches for a string in array values
function arraysearch($arra1, $search)
{
// Reset the array pointer to the beginning
reset($arra1);
// Iterate through the array using a while loop
while (list($key, $val) = each($arra1))
{
// Check if the search string is found (case-insensitive) in the current array value
if (preg_match("/$search/i", $val))
{
// Display a message indicating the search string is found in the current key
echo $search . " has found in " . $key . "\n";
}
else
{
// Display a message indicating the search string is not found in the current key
echo $search . " has not found in " . $key . "\n";
}
}
}
// Define an associative array of exercises
$exercises = array("part1" => "PHP array", "part2" => "PHP String", "part3" => "PHP Math");
// Call the arraysearch function to search for "Math" in the values of the exercises array
arraysearch($exercises, "Math");
?>
Output:
Math has not found in part1 Math has not found in part2 Math has found in part3
Flowchart:
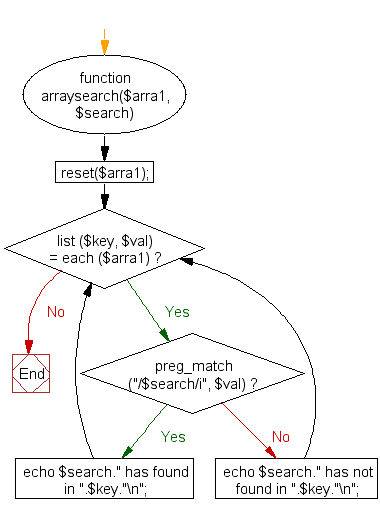
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to get the extension of a file.
Next: Write a PHP program to sort an associative array (alphanumeric with case-sensitive data) by values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics