PHP Array Exercises : Extension of a file
PHP Array: Exercise-32 with Solution
Write a PHP program to get the extension of a file.
Sample Solution:
PHP Code:
<?php
// Define a function named file_extension that takes a file path as an argument
function file_extension($str1){
// Replace backslashes with an empty string in the given path
$str1 = implode("", explode("\\", $str1));
// Explode the path using the dot as a delimiter to extract the file extension
$str1 = explode(".", $str1);
// Convert the file extension to lowercase and return it
$str1 = strtolower(end($str1));
return $str1;
}
// Define a file path 'example.txt'
$file = 'example.txt';
// Call the file_extension function and display the result
echo "\n" . file_extension($file) . "\n";
?>
Output:
txt
Flowchart:
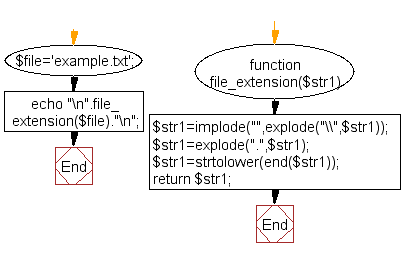
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to get the index of the highest value in an associative array.
Next: Write a PHP function to search a specified value within the values of an associative array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/php-array-exercise-32.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics