PHP Array Exercises : Create a letter range with arbitrary length
Write a PHP program to create a letter range with arbitrary length.
Sample Solution:
PHP Code:
<?php
// Define a function named letter_range that takes a length as input
function letter_range($length)
{
// Initialize an empty array to store the result
$data_range = array();
// Create an array of uppercase letters from 'A' to 'Z'
$letters = range('A', 'Z');
// Iterate through the specified length
for($i=0; $i<$length; $i++)
{
// Calculate the starting position for each iteration
$position = $i * 26;
// Iterate through the letters and generate combinations
foreach($letters as $ii => $letter)
{
// Increment the position
$position++;
// Check if the position is within the specified length
if($position <= $length)
// Add the generated combination to the result array
$data_range[] = ($position > 26 ? $data_range[$i-1] : '').$letter;
}
}
// Return the array containing the generated letter combinations
return $data_range;
}
// Test the function with a length of 7 and print the result
print_r(letter_range(7));
?>
Output:
Array ( [0] => A [1] => B [2] => C [3] => D [4] => E [5] => F [6] => G )
Flowchart:
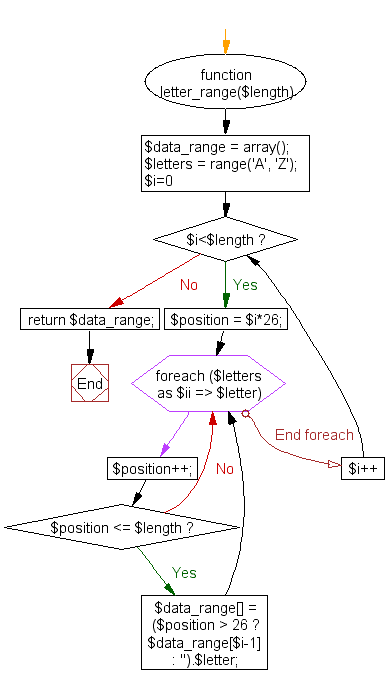
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to generate an array with a range taken from a string.
Next: Write a PHP program to get the index of the highest value in an associative array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.