PHP Array Exercises : Sort entity letters
PHP Array: Exercise-25 with Solution
Write a PHP function to sort entity letters.
Sample Solution:
PHP Code:
<?php
// Define a function to sort an array of entities
function entity_sort($my_array) {
// Iterate through each element in the array
foreach ($my_array as &$element) {
// Check if the element is not empty
if (!empty($element)) {
// Prepend the element with its first character
$element = $element[0] . $element;
}
}
// Sort the modified array
sort($my_array);
// Iterate through each element in the array
foreach ($my_array as &$element) {
// Remove the first character from each element
$element = substr($element, 1);
}
// Return the sorted array
return $my_array;
}
// Create an array containing space, "&", and ">"
$arr = array(" ", "<", "&");
// Call the entity_sort function and print the result
print_r(entity_sort($arr));
?>
Output:
Array ( [0] => [1] => & [2] => < )
Flowchart:
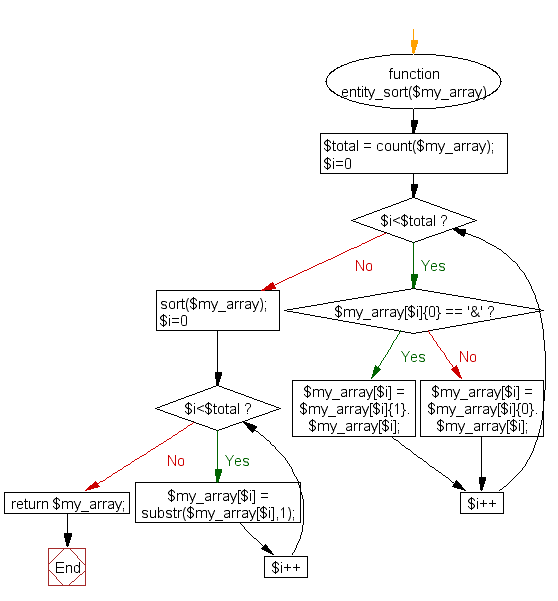
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to sort an array using case-insensitive natural ordering.
Next: Write a PHP function to shuffle an associative array, preserving key, value pairs.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/php-array-exercise-25.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics