PHP Array Exercises : Sort a multi-dimensional array set by a specific key
PHP Array: Exercise-23 with Solution
Write a PHP program to sort a multi-dimensional array set by a specific key.
Sample Solution:
PHP Code:
<?php
// Define a function named 'column_Sort' for sorting a multi-dimensional array based on a specified column
function column_Sort($unsorted, $column) {
// Create a copy of the unsorted array to be sorted
$sorted = $unsorted;
// Iterate through the array using bubble sort algorithm
for ($i = 0; $i < sizeof($sorted) - 1; $i++) {
for ($j = 0; $j < sizeof($sorted) - 1 - $i; $j++) {
// Compare values in the specified column and swap if necessary
if ($sorted[$j][$column] > $sorted[$j + 1][$column]) {
$tmp = $sorted[$j];
$sorted[$j] = $sorted[$j + 1];
$sorted[$j + 1] = $tmp;
}
}
}
// Return the sorted array
return $sorted;
}
// Initialize a multi-dimensional array $my_array with sample data
$my_array = array();
$my_array[0]['name'] = 'Sana';
$my_array[0]['email'] = '[email protected]';
$my_array[0]['phone'] = '111-111-1234';
$my_array[0]['country'] = 'USA';
$my_array[1]['name'] = 'Robin';
$my_array[1]['email'] = '[email protected]';
$my_array[1]['phone'] = '222-222-1235';
$my_array[1]['country'] = 'UK';
$my_array[2]['name'] = 'Sofia';
$my_array[2]['email'] = '[email protected]';
$my_array[2]['phone'] = '333-333-1236';
$my_array[2]['country'] = 'India';
// Print the sorted array based on the 'name' column
print_r(column_Sort($my_array, 'name'));
?>
Output:
Array ( [0] => Array ( [name] => Robin [email] => [email protected] [phone] => 222-222-1235 [country] => UK ) [1] => Array ( [name] => Sana [email] => [email protected] [phone] => 111-111-1234 [country] => USA ) [2] => Array ( [name] => Sofia [email] => [email protected] [phone] => 333-333-1236 [country] => India ) )
Flowchart:
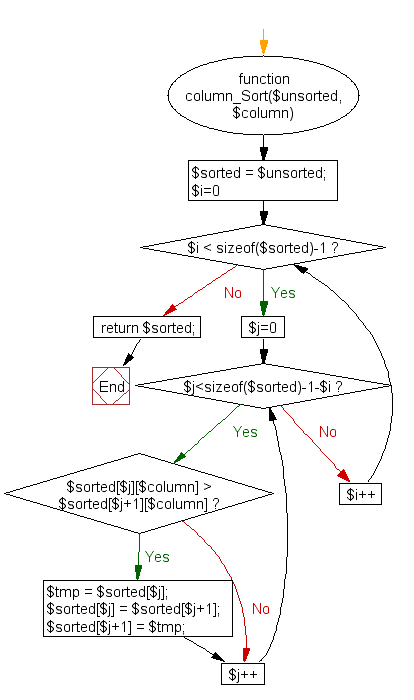
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to sort the following array by the day (page_id) and username.
Next: Write a PHP script to sort an array using case-insensitive natural ordering.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics