PHP Array Exercises : Sort a specified array by the day and username
22. Sort Array by Day and Username
Write a PHP script to sort the following array by the day (page_id) and username.
Sample Solution:
PHP Code:
<?php
// Initialize a multidimensional array $arra with transaction_id and user_name values
$arra[0]["transaction_id"] = "2025731470";
$arra[1]["transaction_id"] = "2025731450";
$arra[2]["transaction_id"] = "1025731456";
$arra[3]["transaction_id"] = "1025731460";
$arra[0]["user_name"] = "Sana";
$arra[1]["user_name"] = "Illiya";
$arra[2]["user_name"] = "Robin";
$arra[3]["user_name"] = "Samantha";
// Define a function convert_timestamp to convert timestamp to date
function convert_timestamp($timestamp){
$limit = date("U");
$limiting = $timestamp - $limit;
return date("Ymd", mktime(0, 0, $limiting));
}
// Define a comparison function cmp for sorting based on transaction_id and user_name
function cmp($a, $b) {
$l = convert_timestamp($a["transaction_id"]);
$k = convert_timestamp($b["transaction_id"]);
if ($k == $l) {
return strcmp($a["user_name"], $b["user_name"]);
} else {
return strcmp($k, $l);
}
}
// Use usort to sort the array $arra using the comparison function cmp
usort($arra, "cmp");
// Print the sorted information
while (list ($key, $value) = each($arra)) {
echo "\$arra[$key]: ";
echo $value["transaction_id"];
echo " user_name: ";
echo $value["user_name"];
echo "\n";
}
?>
Output:
$arra[0]: 2025731450 user_name: Illiya $arra[1]: 2025731470 user_name: Sana $arra[2]: 1025731456 user_name: Robin $arra[3]: 1025731460 user_name: Samantha
Flowchart:
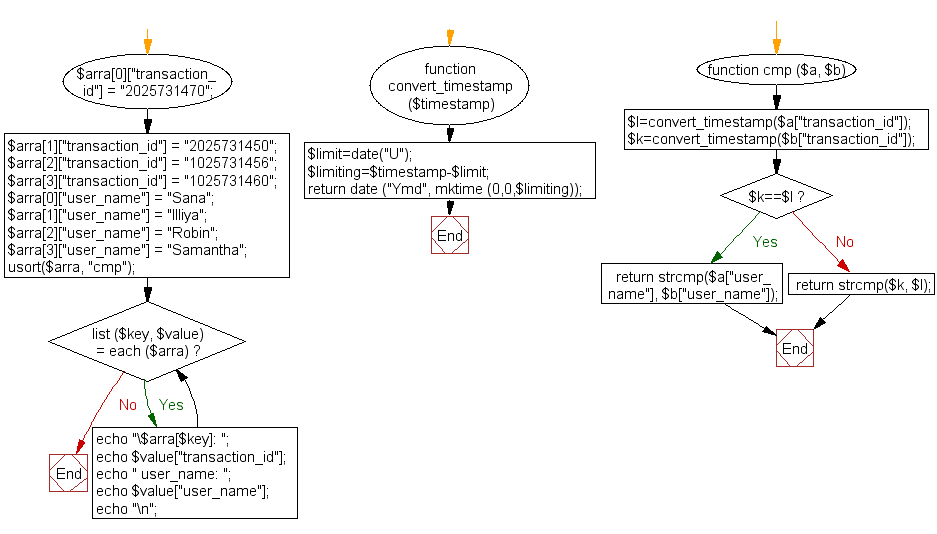
For more Practice: Solve these Related Problems:
- Write a PHP script to sort a multi-dimensional array by two keys: first by day (page_id) and then by username in ascending order.
- Write a PHP function that accepts an array containing day and username fields and outputs the sorted array based on both keys.
- Write a PHP program to group an array by day and then sort the groups alphabetically by username.
- Write a PHP script to combine sorting functions to order a multi-dimensional array by day first and then by username using a custom comparison callback.
Go to:
PREV : Sort Subnets.
NEXT : Sort Multi-Dimensional Array by Specific Key.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.