PHP Array Exercises : Function to sort subnets
Write a PHP function to sort subnets.
Sample Solution:
PHP Code:
<?php
// Define a function named 'sort_subnets' for custom sorting of IP subnets
function sort_subnets ($x, $y) {
// Split IP subnets into arrays of octets
$x_arr = explode('.', $x);
$y_arr = explode('.', $y);
// Iterate through each octet and compare values
foreach (range(0,3) as $i) {
// If the current octet in $x is less than the current octet in $y, return -1 (indicating $x comes first)
if ( $x_arr[$i] < $y_arr[$i] ) {
return -1;
}
// If the current octet in $x is greater than the current octet in $y, return 1 (indicating $y comes first)
elseif ( $x_arr[$i] > $y_arr[$i] ) {
return 1;
}
}
// If all octets are equal, return -1 (indicating $x comes first)
return -1;
}
// Define an array of IP subnets
$subnet_list = array(
'192.169.12',
'192.167.11',
'192.169.14',
'192.168.13',
'192.167.12',
'122.169.15',
'192.167.16'
);
// Use 'usort' function to sort the array of IP subnets using the 'sort_subnets' custom sorting function
usort($subnet_list, 'sort_subnets');
// Print the sorted array of IP subnets
print_r($subnet_list);
?>
Output:
Array ( [0] => 122.169.15 [1] => 192.167.11 [2] => 192.167.12 [3] => 192.167.16 [4] => 192.168.13 [5] => 192.169.12 [6] => 192.169.14 )
Flowchart:
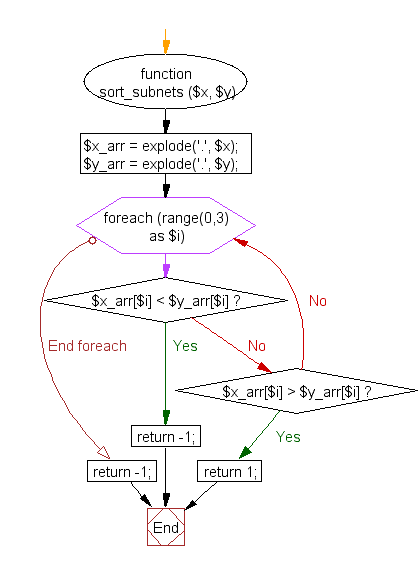
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP function to sort an array according to another array acting as a priority list.
Next: Write a PHP script to sort the following array by the day (page_id) and username.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.