PHP Array Exercises : Return the lowest integer (not 0) from a list of numbers
17. Lowest Non-zero Integer Function
Write a PHP function that returns the lowest integer that is not 0.
Sample Solution:
PHP Code:
<?php
// Define a function named 'min_values_not_zero' that takes an array of values as input
function min_values_not_zero(Array $values)
{
// Use 'array_map' to convert each element to an integer, 'array_diff' to exclude zero, and 'min' to find the minimum value
return min(array_diff(array_map('intval', $values), array(0)));
}
// Call the 'min_values_not_zero' function with an example array and print the result
print_r(min_values_not_zero(array(-1, 0, 1, 12, -100, 1)) . "\n");
?>
Output:
-100
Flowchart:
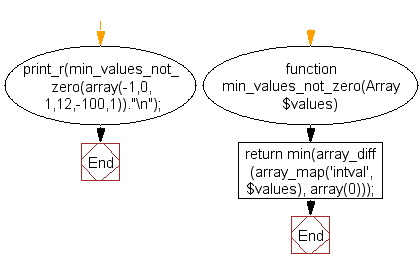
For more Practice: Solve these Related Problems:
- Write a PHP function to scan an array of integers and return the smallest integer that is not zero, ignoring zeros.
- Write a PHP script to iterate through an array, filtering out zeros, and then compute the minimum of the remaining values.
- Write a PHP program to sort an array and select the first element that is not equal to zero.
- Write a PHP function that uses array_filter to remove zeros from an array, then applies min() to find the lowest positive number.
Go to:
PREV : Get the Largest Key in an Array.
NEXT : Floor Decimal with Precision.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.