PHP Array Exercises : Generate unique random numbers within a range
Write a PHP script to generate unique random numbers within a range.
Sample Range : (11, 20)
Sample Solution:
PHP Code:
<?php
// Generate an array containing numbers from 11 to 20 using 'range'
$n = range(11, 20);
// Shuffle the elements of the array using 'shuffle'
shuffle($n);
// Use a 'for' loop to iterate 10 times
for ($x = 0; $x < 10; $x++)
{
// Echo the current element of the shuffled array followed by a space
echo $n[$x] . ' ';
}
// Echo a newline character for better formatting
echo "\n";
?>
Output:
17 19 13 15 20 14 12 18 11 16
Flowchart:
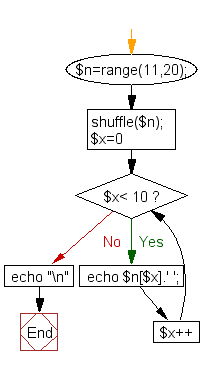
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to get the shortest/longest string length from an array.
Next: Write a PHP script to get the largest key in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics