PHP Array Exercises : Merge two arrays
PHP Array: Exercise-11 with Solution
Write a PHP program to merge (by index) the following two arrays.
Sample arrays :
$array1 = array(array(77, 87), array(23, 45));
$array2 = array("w3resource", "com");
Sample Solution:
PHP Code:
<?php
// Define a multidimensional array $array1
$array1 = array(array(77, 87), array(23, 45));
// Define a simple array $array2
$array2 = array("w3resource", "com");
// Define a function named 'merge_arrays_by_index' that merges elements based on their indexes
function merge_arrays_by_index($x, $y)
{
// Create an empty temporary array
$temp = array();
// Add the first element $x to the temporary array
$temp[] = $x;
// Check if $y is a scalar value
if (is_scalar($y))
{
// If $y is scalar, add it directly to the temporary array
$temp[] = $y;
}
else
{
// If $y is an array, iterate through its key-value pairs and add values to the temporary array
foreach ($y as $k => $v)
{
$temp[] = $v;
}
}
// Return the temporary array
return $temp;
}
// Output HTML preformatted text
echo '<pre>';
// Use array_map to apply 'merge_arrays_by_index' to corresponding elements of $array2 and $array1
print_r(array_map('merge_arrays_by_index', $array2, $array1));
?>
Output:
<pre>Array ( [0] => Array ( [0] => w3resource [1] => 77 [2] => 87 ) [1] => Array ( [0] => com [1] => 23 [2] => 45 ) )
Flowchart:
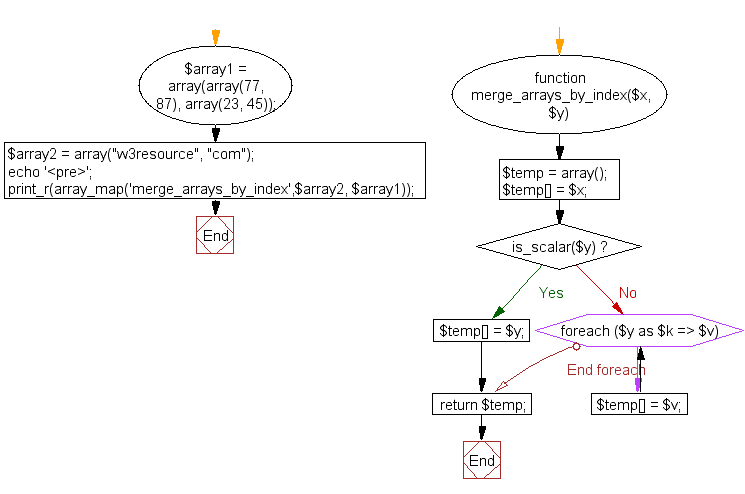
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to sort an array of positive integers using the Bead-Sort Algorithm.
Next: Write a PHP function to change the specified array's all values to upper or lower case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics