PHP class hierarchy for library system
Write a PHP a class hierarchy for a library system, including classes like 'LibraryItem', 'Book', 'DVD', etc. Implement appropriate properties and methods for each class.
Sample Solution:
PHP Code :
<?php
class LibraryItem {
protected $title;
protected $author;
protected $year;
public function __construct($title, $author, $year) {
$this->title = $title;
$this->author = $author;
$this->year = $year;
}
public function getTitle() {
return $this->title;
}
public function getAuthor() {
return $this->author;
}
public function getYear() {
return $this->year;
}
}
class Book extends LibraryItem {
protected $genre;
public function __construct($title, $author, $year, $genre) {
parent::__construct($title, $author, $year);
$this->genre = $genre;
}
public function getGenre() {
return $this->genre;
}
public function displayDetails() {
echo "Title: " . $this->title . "</br>";
echo "Author: " . $this->author . "</br>";
echo "Year: " . $this->year . "</br>";
echo "Genre: " . $this->genre . "</br>";
}
}
class DVD extends LibraryItem {
protected $duration;
public function __construct($title, $author, $year, $duration) {
parent::__construct($title, $author, $year);
$this->duration = $duration;
}
public function getDuration() {
return $this->duration;
}
public function displayDetails() {
echo "</br>Title: " . $this->title . "</br>";
echo "Author: " . $this->author . "</br>";
echo "Year: " . $this->year . "</br>";
echo "Duration: " . $this->duration . " minutes";
}
}
$book = new Book("Don Quixote", "Miguel de Cervantes", 1605, "Epic novel");
$book->displayDetails();
$dvd = new DVD("The Land Before Time", "Charles Grosvenor", 2010, 150);
$dvd->displayDetails();
?>
Sample Output:
Title: Don Quixote Author: Miguel de Cervantes Year: 1605 Genre: Epic novel Title: The Land Before Time Author: Charles Grosvenor Year: 2010 Duration: 150 minutes
Explanation:
In the above exercise -
- The "LibraryItem" class serves as the base class for all library items and defines common properties like $title, $author, and $year. It also provides getter methods to access these properties.
- The "Book" class extends the LibraryItem class and adds an additional property $genre. It has its own constructor to initialize the properties, and a getGenre() method to access the genre. It also overrides the displayDetails() method to display specific details of a book.
- The "DVD" class also extends the LibraryItem class and adds an additional property $duration. It has its own constructor and a getDuration() method to access the duration. It also overrides the displayDetails() method to display specific details of a DVD.
Flowchart:
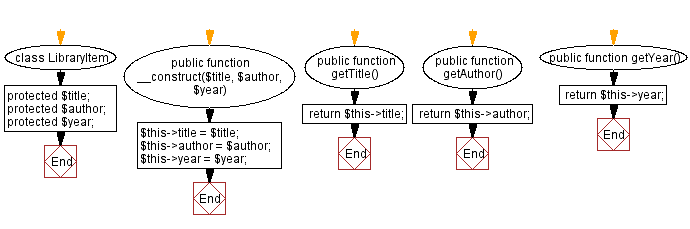
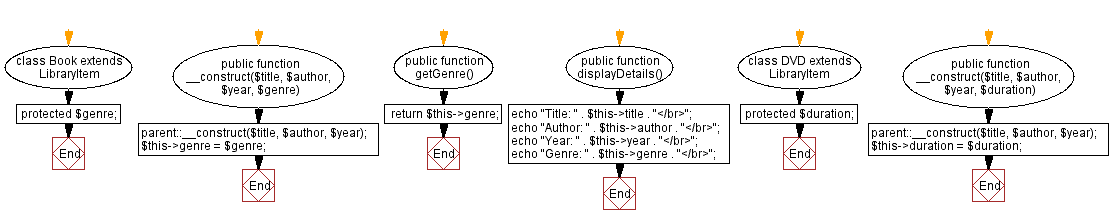
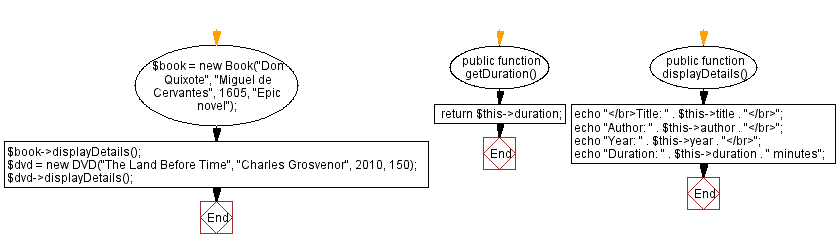
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Display vehicle details.
Next: Retrieve and display PHP cookie value for a given user.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics