PHP Class Shape: Abstract method and subclass implementation
Write a PHP class called 'Shape' with an abstract method 'calculateArea()'. Create two subclasses, 'Triangle' and 'Rectangle', that implement the 'calculateArea()' method.
Sample Solution:
PHP Code :
<?php
abstract class Shape {
abstract public function calculateArea();
}
class Triangle extends Shape {
private $base;
private $height;
public function __construct($base, $height) {
$this->base = $base;
$this->height = $height;
}
public function calculateArea() {
return 0.5 * $this->base * $this->height;
}
}
class Rectangle extends Shape {
private $length;
private $width;
public function __construct($length, $width) {
$this->length = $length;
$this->width = $width;
}
public function calculateArea() {
return $this->length * $this->width;
}
}
$triangle = new Triangle(5, 7);
echo "Triangle Area: " . $triangle->calculateArea() . "</br>";
$rectangle = new Rectangle(4, 6);
echo "Rectangle Area: " . $rectangle->calculateArea() . "</br>";
?>
Sample Output:
Triangle Area: 17.5 Rectangle Area: 24
Flowchart:
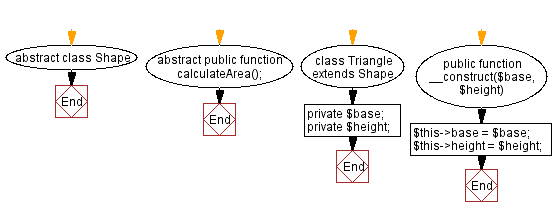
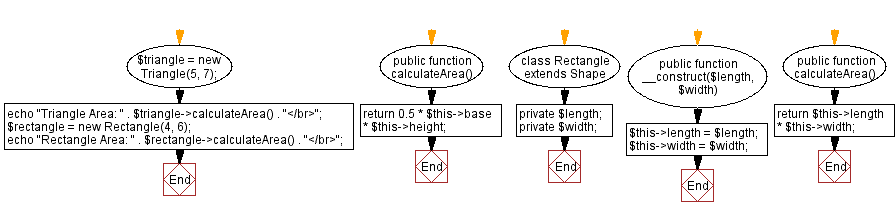
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Area and circumference calculation.
Next: Resizing functionality in the square class.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics