PHP class for circle: Area and circumference calculation
Write a PHP class called 'Circle' that has a radius property. Implement methods to calculate the circle's area and circumference.
Sample Solution:
PHP Code :
<?php
class Circle {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function getArea() {
return pi() * pow($this->radius, 2);
}
public function getCircumference() {
return 2 * pi() * $this->radius;
}
}
$circle = new Circle(7);
echo "Area: " . $circle->getArea() . "</br>";
echo "Circumference: " . $circle->getCircumference() . "</br>";
?>
Sample Output:
Area: 153.9380400259 Circumference: 43.982297150257
Explanation:
In the above exercise -
- The "Circle" class has one private property: $radius, which represents the circle radius.
- The constructor method __construct() initializes the circle radius.
- The getArea() method calculates and returns the area of the circle using the formula: π * r^2, where π is a constant value obtained from the pi() function, and r is the radius of the circle.
- The getCircumference() method calculates and returns the circle circumference using the formula: 2*π*r.
Flowchart:
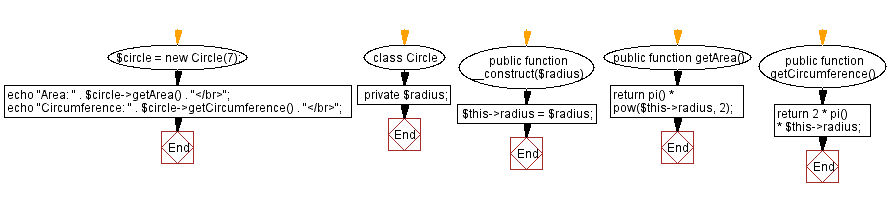
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Area and perimeter calculation.
Next: Abstract method and subclass implementation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.