PHP OOP: Shopping cart class
Write a PHP class called 'ShoppingCart' with properties like 'items' and 'total'. Implement methods to add items to the cart and calculate the total cost.
Sample Solution:
PHP Code :
<?php
class ShoppingCart {
private $items;
private $total;
public function __construct() {
$this->items = [];
$this->total = 0;
}
public function addItem($item, $price) {
$this->items[$item] = $price;
$this->total += $price;
}
public function getTotal() {
return $this->total;
}
}
$cart = new ShoppingCart();
$cart->addItem("Product 1", 20);
$cart->addItem("Product 2", 30);
$cart->addItem("Product 3", 10);
$total = $cart->getTotal();
echo "Total cost: $" . $total;
?>
Sample Output:
Total cost: $60
Explanation:
In the above exercise -
- The "ShoppingCart" class has two private properties: $items and $total. $items is an associative array that stores the items in the cart as keys and their corresponding prices as values. $total keeps track of the total cost of all items in the cart.
- The constructor method __construct() initializes the $items array as an empty array and sets the $total to 0.
- The addItem($item, $price) method takes $item and its corresponding $price as parameters. It adds the item and its price to the $items array and increments the $total by the price.
- The getTotal() method retrieves the total cost of the items in the cart.
Flowchart:
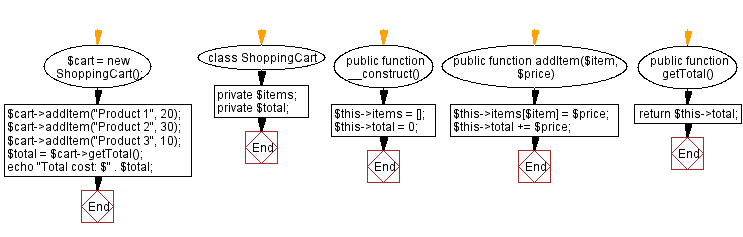
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Perform Arithmetic operations.
Next: Singleton design pattern implementation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.