PHP class for file operations
Write a PHP class called 'File' with properties like 'name' and 'size'. Implement a static method to calculate the total size of multiple files.
Sample Solution:
PHP Code :
<?php
class File {
public $name;
public $size;
public function __construct($name, $size) {
$this->name = $name;
$this->size = $size;
}
public static function calculateTotalSize($files) {
$totalSize = 0;
foreach ($files as $file) {
if ($file instanceof File) {
$totalSize += $file->size;
}
}
return $totalSize;
}
}
$file1 = new File("file1.txt", 1000);
$file2 = new File("file2.txt", 2000);
$file3 = new File("file3.txt", 1500);
$files = [$file1, $file2, $file3];
$totalSize = File::calculateTotalSize($files);
echo "Total size of files: " . $totalSize . " bytes";
?>
Sample Output:
Total size of files: 4500 bytes
Explanation:
In the above exercise -
- The "File" class has two public properties: $name and $size, which represent the name and size of a file, respectively.
- The constructor method __construct() is used to initialize the values of the properties when creating a new instance of the File class.
- The calculateTotalSize() method is a static method that accepts an array of files as a parameter. It iterates through each file in the array, checks if it is an instance of the File class, and adds up the files' sizes.
- The method returns the total size.
Flowchart:
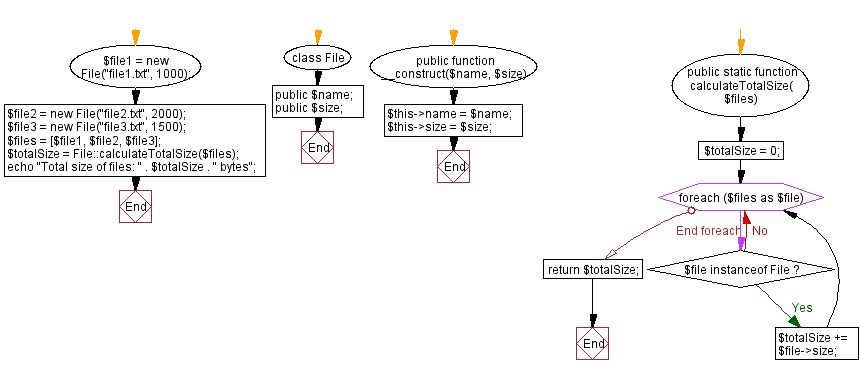
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP class for mathematical calculations.
Next: Perform Arithmetic operations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics