PHP class with comparable interface
12. Product Class with Comparable Interface
Write a class called 'Product' with properties like 'name' and 'price'. Implement the 'Comparable' interface to compare products based on their prices.
Sample Solution:
PHP Code :
<?php
interface Comparable {
public function compareTo($other);
}
class Product implements Comparable {
private $name;
private $price;
public function __construct($name, $price) {
$this->name = $name;
$this->price = $price;
}
public function getName() {
return $this->name;
}
public function getPrice() {
return $this->price;
}
public function compareTo($other) {
if ($other instanceof Product) {
if ($this->price < $other->getPrice()) {
return -1;
} elseif ($this->price > $other->getPrice()) {
return 1;
} else {
return 0;
}
} else {
throw new Exception("Invalid comparison object.");
}
}
}
$product1 = new Product("Desktop", 1200);
$product2 = new Product("Laptop", 1000);
$result = $product1->compareTo($product2);
if ($result < 0) {
echo $product1->getName() . " is cheaper than " . $product2->getName() . "</br>";
} elseif ($result > 0) {
echo $product1->getName() . " is more expensive than " . $product2->getName() . "</br>";
} else {
echo $product1->getName() . " and " . $product2->getName() . " have the same price.</br>";
}
?>
Sample Output:
Desktop is more expensive than Laptop
Explanation:
In the above exercise -
- The Comparable interface declares a single method compareTo() that accepts another object for comparison.
- The "Product" class implements the Comparable interface and defines the compareTo() method.
- The Product class has two private properties: $name and $price, representing the product's name and price.
- The constructor method __construct() is used to initialize the values of the properties when creating a new instance of the Product class.
- The getName() and getPrice() methods retrieve name and price, respectively.
- The compareTo() method compares two Product objects based on their prices. It returns -1, 0, or 1 indicating whether the current object is less than, equal to, or greater than the compared object.
Flowchart:
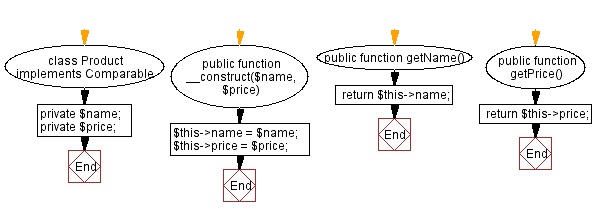
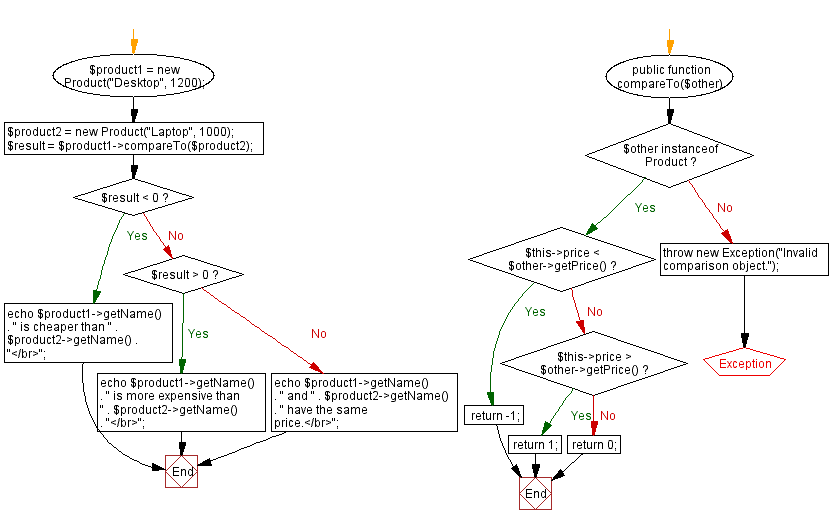
For more Practice: Solve these Related Problems:
- Write a PHP class Product implementing a Comparable-like interface to allow products to be sorted by price.
- Write a PHP script to create an array of Product objects, then sort them in ascending order by their price property.
- Write a PHP function to compare two Product objects and return 1, -1, or 0 based on price difference.
- Write a PHP program to display the details of the most expensive product from an array of Product objects after sorting.
Go to:
PREV : Employee Extending Person.
NEXT : Logger Class with Static LogCount.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.