PHP class inheritance with extended class
PHP OOP: Exercise-11 with Solution
Write a class called 'Employee' that extends the 'Person' class and adds properties like 'salary' and 'position'. Implement methods to display employee details.
Sample Solution:
PHP Code :
<?php
class Person {
protected $name;
protected $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function __toString() {
return "Name: " . $this->name . "\n" .
"Age: " . $this->age . "\n";
}
}
class Employee extends Person {
private $salary;
private $position;
public function __construct($name, $age, $salary, $position) {
parent::__construct($name, $age);
$this->salary = $salary;
$this->position = $position;
}
public function getSalary() {
return $this->salary;
}
public function getPosition() {
return $this->position;
}
public function displayDetails() {
echo "Name: " . $this->name . "</br>";
echo "Age: " . $this->age . "</br>";
echo "Salary: " . $this->salary . "</br>";
echo "Position: " . $this->position . "</br>";
}
}
$employee = new Employee("Pratik Octavius", 33, 6000, "Manager");
$employee->displayDetails();
?>
Sample Output:
Name: Pratik Octavius Age: 33 Salary: 6000 Position: Manager
Flowchart:
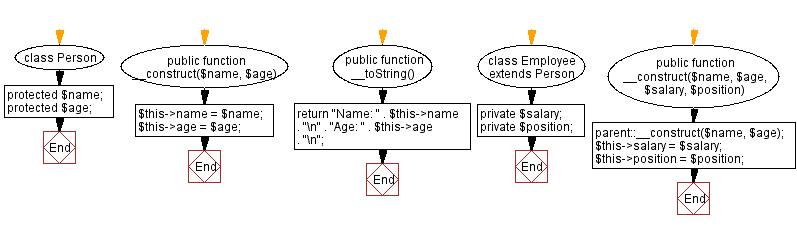
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Class with magic method.
Next: PHP class with comparable interface.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics