PHP class for rectangle: Area and perimeter calculation
PHP OOP: Exercise-1 with Solution
Write a PHP class 'Rectangle' that has properties for length and width. Implement methods to calculate the rectangle's area and perimeter.
Sample Solution:
PHP Code :
<?php
class Rectangle {
private $length;
private $width;
public function __construct($length, $width) {
$this->length = $length;
$this->width = $width;
}
public function getArea() {
return $this->length * $this->width;
}
public function getPerimeter() {
return 2 * ($this->length + $this->width);
}
}
$rectangle = new Rectangle(12, 9);
echo "Area: " . $rectangle->getArea() . "</br>";
echo "Perimeter: " . $rectangle->getPerimeter() . "</br>";
?>
Sample Output:
Area: 108 Perimeter: 42
Explanation:
In the above exercise -
- The "Rectangle" class has two private properties: $length and $width, which represent the rectangle's dimensions.
- The constructor method __construct() initializes the rectangle's length and width.
- The getArea() method calculates and returns the rectangle's area by multiplying its length and width.
- The getPerimeter() method calculates and returns the rectangle perimeter using the formula: 2 * (length + width).
Flowchart:
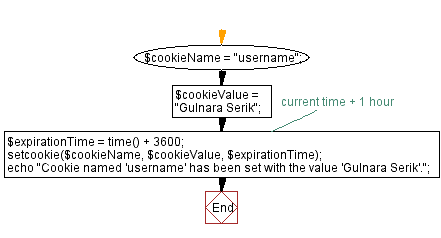
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP OOP Exercises Home.
Next: Area and circumference calculation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/oop/php-oop-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics