PHP script to rename a file
Write a PHP script to rename a file.
Sample Solution:
PHP Code :
<?php
$oldFilename = "i:/example.txt";
$newFilename = "i:/new_example.txt";
try {
if (file_exists($oldFilename)) {
if (rename($oldFilename, $newFilename)) {
echo "File renamed successfully.";
} else {
throw new Exception("Error renaming the file.");
}
} else {
throw new Exception("File does not exist.");
}
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
File renamed successfully.
Explanation:
In the above exercise -
- We have two variables: $oldFilename holds the current name of the file you want to rename, and $newFilename holds the desired new name of the file.
- Inside the try block, we first check if the old file exists using file_exists(). If it exists, we use the rename() function to rename the file. If the renaming operation is successful, we display a success message. If the renaming fails, we throw an exception with the error message "Error renaming the file."
- If the old file does not exist, we throw an exception with the error message "File does not exist."
- In the catch block, we catch the exception and display an error message using $e->getMessage().
Flowchart:
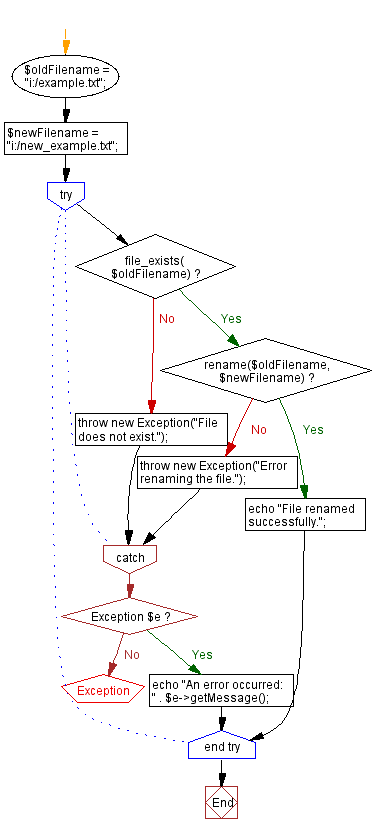
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP program to read and display CSV data.
Next: PHP function to copy a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics