PHP script to count lines in a text file
3. Count the number of lines in a text file
Write a PHP script to count the number of lines in a text file.
Sample Solution:
PHP Code :
<?php
$filename = "i:/test.txt";
try {
$lineCount = 0;
$fileHandle = fopen($filename, 'r');
if ($fileHandle === false) {
throw new Exception("Error opening the file.");
}
while (!feof($fileHandle)) {
$line = fgets($fileHandle);
if ($line !== false) {
$lineCount++;
}
}
fclose($fileHandle);
echo "Number of lines in the file: " . $lineCount;
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
Number of lines in the file: 5
Explanation:
In the above exercise -
- We have a $filename variable that holds the name of the text file for which we want to count the lines.
- Inside the try block, we initialize a $lineCount variable to 0, which stores the number of lines.
- We open the file using fopen() with the 'r' mode to read the file.
- If there is an error opening the file, we throw an exception with the error message "Error opening the file."
- We then enter a loop using feof() to check if we have reached the end of the file. Inside the loop, we use fgets() to read each line from the file. If a line is successfully read ($line !== false), we increment the $lineCount variable.
- After counting the lines, we close the file using fclose().
- Finally, we display the number of lines in the file using the echo statement.
Flowchart:
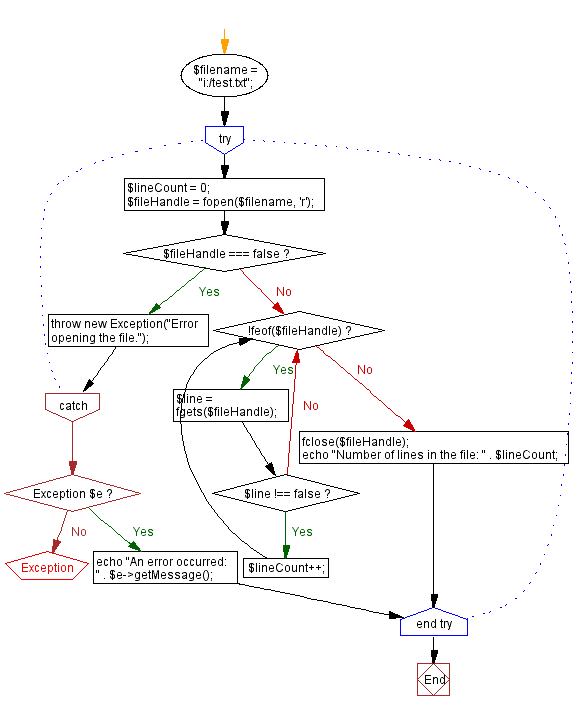
For more Practice: Solve these Related Problems:
- Write a PHP function that checks for multiple file paths and returns an array of files that exist.
- Write a PHP script to check if a file exists and is accessible by a specific user using permissions.
- Write a PHP function to recursively check if a file exists in nested directories.
- Write a PHP script to verify if a file exists and validate its MIME type before displaying its contents.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP function to check file existence by path.
Next: PHP function to write string to file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.