PHP: Read and extract data from an XML file
PHP File Handling: Exercise-16 with Solution
Write a PHP program that reads an XML file and extracts specific data from it.
books.xml
<?xml version="1.0"?>
<catalog>
<book id="bk101">
<author>Gambardella, Matthew</author>
<title>XML Developer's Guide</title>
<genre>Computer</genre>
<price>44.95</price>
<publish_date>2000-10-01</publish_date>
<description>An in-depth look at creating applications
with XML.</description>
</book>
<book id="bk102">
<author>Ralls, Kim</author>
<title>Midnight Rain</title>
<genre>Fantasy</genre>
<price>5.95</price>
<publish_date>2000-12-16</publish_date>
<description>A former architect battles corporate zombies,
an evil sorceress, and her own childhood to become queen
of the world.</description>
</book>
<book id="bk103">
<author>Corets, Eva</author>
<title>Maeve Ascendant</title>
<genre>Fantasy</genre>
<price>5.95</price>
<publish_date>2000-11-17</publish_date>
<description>After the collapse of a nanotechnology
society in England, the young survivors lay the
foundation for a new society.</description>
</book>
<book id="bk104">
<author>Corets, Eva</author>
<title>Oberon's Legacy</title>
<genre>Fantasy</genre>
<price>5.95</price>
<publish_date>2001-03-10</publish_date>
<description>In post-apocalypse England, the mysterious
agent known only as Oberon helps to create a new life
for the inhabitants of London. Sequel to Maeve
Ascendant.</description>
</book>
<book id="bk105">
<author>Corets, Eva</author>
<title>The Sundered Grail</title>
<genre>Fantasy</genre>
<price>5.95</price>
<publish_date>2001-09-10</publish_date>
<description>The two daughters of Maeve, half-sisters,
battle one another for control of England. Sequel to
Oberon's Legacy.</description>
</book>
</catalog>
Sample Solution:
PHP Code :
<?php
$filename = "i:/books.xml";
$desiredAuthor = "Ralls, Kim";
echo "Search for the author: " . $desiredAuthor;
try {
$xml = simplexml_load_file($filename);
// Iterate over book elements and extract specific data based on condition
foreach ($xml->book as $book) {
$author = $book->author;
if ($author == $desiredAuthor) {
$title = $book->title;
$price = $book->price;
$genre = $book->genre;
// Display the extracted data
echo "</br>Title: " . $title . "\n";
echo "</br>Genre: " . $genre . "\n";
echo "</br>Price: " . $price . "\n";
}
}
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
Search for the author: Ralls, Kim Title: Midnight Rain Genre: Fantasy Price: 5.95
Explanation:
In the above exercise -
- The $filename variable holds the name of the XML file we want to read and extract data from.
- Inside the try block, we use the simplexml_load_file() function to load the XML file and parse it into a SimpleXMLElement object.
- In the event that there is an error loading the file or parsing the XML, we throw an exception with the error message "An error occurred."
- We then extract specific data from the XML using object notation (->) and the XML element names.
- Finally, we display the extracted data using the echo statement.
Flowchart:
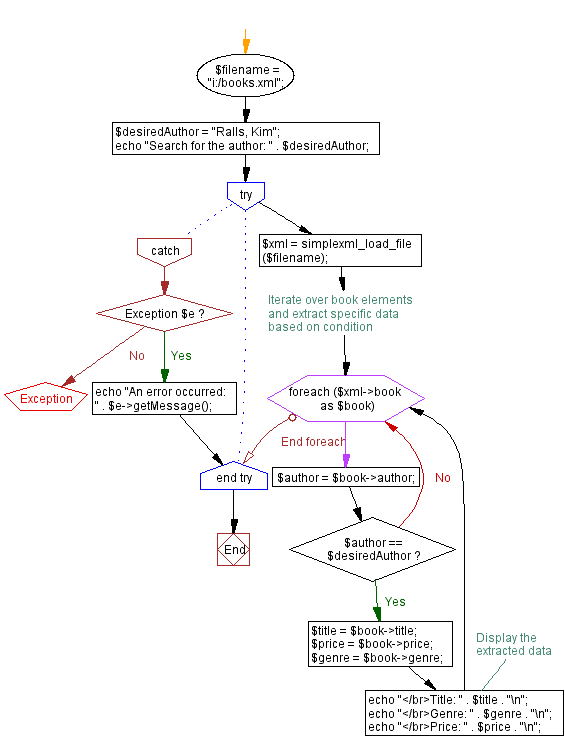
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Read and display file contents.
Next: Read and convert to an associative array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/file/php-file-handling-exercise-16.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics