PHP Exception Handling: Try-Catch blocks for error messages
4. Handling Different Exception Types
Write a PHP script that uses try-catch blocks to handle different types of exceptions and display appropriate error messages.
Sample Solution:
PHP Code :
<?php
try {
// Code that may throw different types of exceptions
$file = "test.txt";
if (!file_exists($file)) {
throw new RuntimeException("File does not exist.");
}
$handle = fopen($file, "r");
if (!$handle) {
throw new Exception("Error opening file.");
}
// Perform some file operations
// ...
fclose($handle);
} catch (RuntimeException $e) {
// Handle RuntimeException
echo "RuntimeException: " . $e->getMessage();
} catch (Exception $e) {
// Handle generic Exception
echo "Exception: " . $e->getMessage();
} finally {
// Clean up resources or perform final actions
echo "Script execution completed.";
}
?>
Sample Output:
RuntimeException: File does not exist.Script execution completed.
Explanation:
In the above exercise,
- We have a try block that contains code that throws different types of exceptions. We first check if a file exists using the file_exists() function and throw a RuntimeException if the file doesn't exist. Then, we try to open the file using fopen() and throw a generic Exception if the file opening fails.
- The catch blocks catch specific exception types (RuntimeException and Exception) and handle them separately. We display appropriate error messages using $e->getMessage().
- Finally, the finally block is optional and allows you to clean up resources or perform final actions. In this example, we simply display a message indicating script execution completion.
Flowchart:
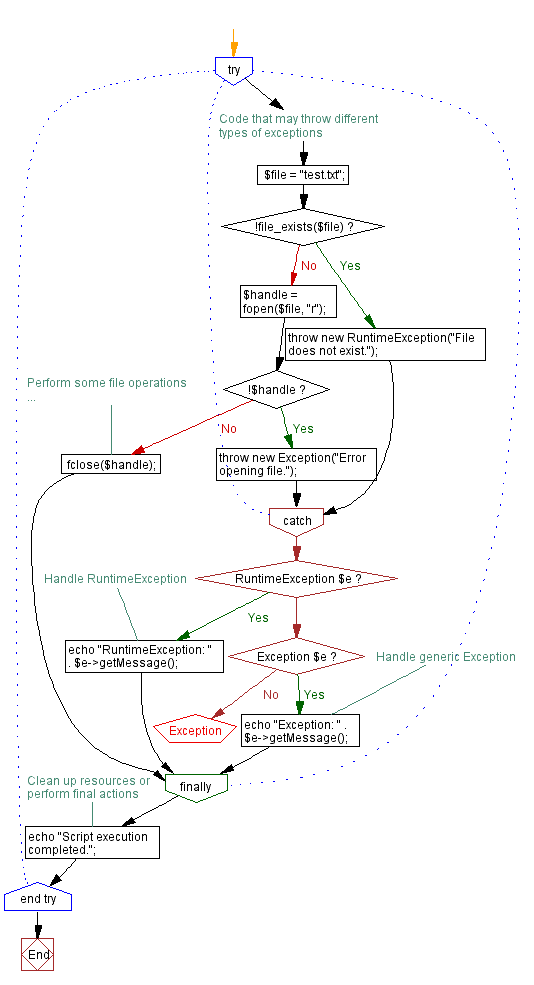
For more Practice: Solve these Related Problems:
- Write a PHP script that uses multiple catch blocks to differentiate between built-in exceptions and custom exceptions thrown within the try block.
- Write a PHP function that deliberately throws different types of exceptions based on input conditions and handles each type separately.
- Write a PHP program that demonstrates catching an exception, re-throwing it, and then catching it again in an outer try-catch structure.
- Write a PHP script to simulate various error scenarios within a single try block and use conditionals in catch blocks to output distinct messages based on the exception type.
Go to:
PREV : Division with Exception Handling.
NEXT : File Read with Custom Exception.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.