Retrieve and display a PHP session variable
5. Retrieve "userid" Session Variable
Write a PHP script to retrieve and display the value of the session variable "userid".
Sample Solution:
PHP Code :
<?php
session_save_path('i:/custom/');
session_start();
if (isset($_SESSION["userid"])) {
$userid = $_SESSION["userid"];
echo "Value of session variable 'userid': " . $userid;
} else {
echo "Session variable 'userid' not found.";
}
?>
Sample Output:
Value of session variable 'userid': 10020
Explanation:
In the above exercise -
- The code starts with session_save_path('i:/custom/'). This line sets a custom path (i:/custom/) for storing session files. You should replace i:/custom/ with the actual path where you want to store session files. This step is optional and allows you to specify a custom session storage location.
- The code then calls session_start(). This function initializes a new or existing session. It enables session variables throughout the script. It must be called before accessing or setting session variables.
- After starting the session, the code checks if the session variable named "userid" is set using isset($_SESSION["userid"]). If the session variable is set, it enters the if block.
- Inside the if block, it retrieves the value of the session variable "userid" using $_SESSION["userid"] and assigns it to the variable $userid.
Flowchart:
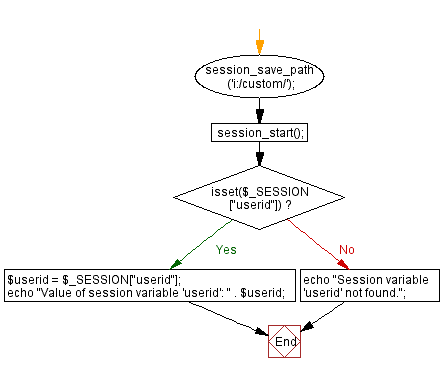
For more Practice: Solve these Related Problems:
- Write a PHP script to start a session and retrieve the "userid" session variable, displaying it on the page.
- Write a PHP function that checks if "userid" exists in the session and prints its value, otherwise outputs a default message.
- Write a PHP program to retrieve "userid" and compare it against a hardcoded value, outputting a verification result.
- Write a PHP script to display the "userid" session variable along with the session ID and last accessed time.
Go to:
PREV : Set "userid" Session Variable.
NEXT : Destroy Session and Unset Variables.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.