PHP Challenges: Compute and return the square root of a given number
Write a PHP program to compute and return the square root of a given number.
Input : 16
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to calculate the square root of a number using the Newton's method
function my_sqrt($n)
{
// Initialize variables $x and $y
$x = $n;
$y = 1;
// Loop until $x is greater than $y
while($x > $y)
{
// Update $x and $y according to Newton's method
$x = ($x + $y) / 2;
$y = $n / $x;
}
// Return the calculated square root
return $x;
}
// Test the my_sqrt function with different inputs and print the results
print_r(my_sqrt(16)."\n");
print_r(my_sqrt(14)."\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- The code defines a function named "my_sqrt()" which calculates the square root of a number using Newton's method. It takes a single parameter '$n', which is the number for which the square root is to be calculated.
- Inside the function:
- It initializes two variables '$x' and '$y' with initial values of '$n' and 1 respectively.
- It enters a "while" loop that continues until "$x" is greater than "$y".
- Within each iteration of the loop, it updates the values of "$x" and "$y" according to Newton's method for finding square roots.
- After the loop, it returns the calculated square root "$x".
- Function Call & Testing:
- The function "my_sqrt()" is called twice with different input values (16 and 14).
- The results of the function calls are printed using print_r(), which displays the calculated square root for each input value.
Sample Output:
4 3.7416573867739
Flowchart:
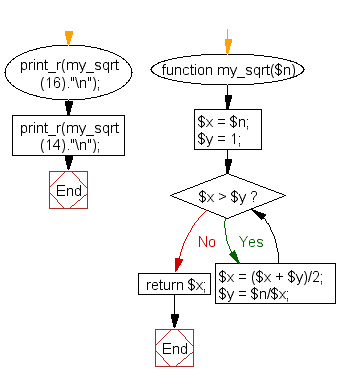
Go to:
PREV : Write a PHP program to find three numbers from an array such that the sum of three consecutive numbers equal to a given number.
NEXT : Write a PHP program to find a single number in an array that doesn't occur twice.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.