PHP Challenges: Find three numbers from an array such that the sum of three consecutive numbers equal to a given number
PHP Challenges - 1: Exercise-7 with Solution
Write a PHP program to find three numbers from an array such that the sum of three consecutive numbers equal to a given number.
Input : (array(2, 7, 7, 1, 8, 2, 7, 8, 7), 16))
Sample Solution :
PHP Code :
<?php
// Function to find triplets in an array whose sum equals a given target
function three_Sum($arr, $target)
{
// Calculate the number of elements in the array minus 2
$count = count($arr) - 2;
// Initialize an empty array to store the result
$result=[];
// Iterate through the array
for ($x = 0; $x < $count; $x++) {
// Check if the sum of the current element and the next two elements equals the target
if ($arr[$x] + $arr[$x+1] + $arr[$x+2] == $target) {
// If true, push the triplet to the result array
array_push($result, "{$arr[$x]} + {$arr[$x+1]} + {$arr[$x+2]} = $target");
}
}
// Return the array of triplets whose sum equals the target
return $result;
}
// Define an array of numbers
$my_array = array(2, 7, 7, 1, 8, 2, 7, 8, 7);
// Test the three_Sum function with the defined array and different target values, and print the result
print_r(three_Sum($my_array, 16));
print_r(three_Sum($my_array, 11));
print_r(three_Sum($my_array, 12));
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "three_Sum()" which takes two parameters: an array '$arr' and a target number '$target'.
- Inside the function:
- It calculates the number of elements in the array minus 2 and stores it in '$count'.
- It initializes an empty array '$result' to store the triplets whose sum equals the target.
- It iterates through the array using a "for" loop.
- For each iteration, it checks if the sum of the current element and the next two elements equals the target.
- If the condition is true, it formats the triplet as a string and pushes it into the '$result' array.
- Finally, it returns the '$result' array containing all the triplets whose sum equals the target.
- Function Call & Testing:
- An array '$my_array' is defined with a set of numbers.
- The "three_Sum()" function is called three times with this array as input and different target sums (16, 11, 12).
- The results of the function calls are printed using "print_r()", which displays the array of triplets whose sum equals the target for each input target sum.
Sample Output:
Array ( [0] => 2 + 7 + 7 = 16 [1] => 7 + 1 + 8 = 16 ) Array ( [0] => 1 + 8 + 2 = 11 ) Array ( )
Flowchart:
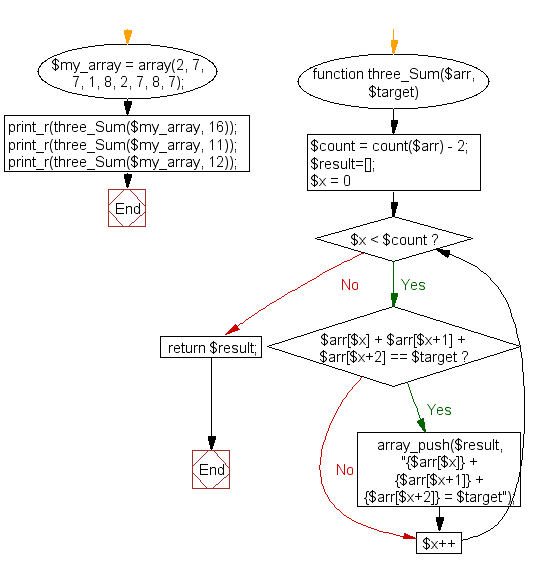
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to find three numbers from an array such that the sum of three consecutive numbers equal to zero.
Next: Write a PHP program to compute and return the square root of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/challenges/1/php-challenges-1-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics