PHP Challenges: Find the single number which occurs odd number of times and other numbers occur even number of times
PHP Challenges - 1: Exercise-25 with Solution
Write a PHP program to find the single number which occurs odd number of times and other numbers occur even number of times.
Input : 4, 5, 4, 5, 2, 2, 3, 3, 2, 4, 4
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to find the element with odd occurrences in an array
function odd_occurrence($arr)
{
// Initialize result variable to store the odd occurrence
$result = 0;
// Traverse the array
foreach ($arr as &$value)
{
// Perform bitwise XOR operation
// Bits that are set in $a or $b but not both are set.
$result = $result ^ $value;
}
// Return the element with odd occurrences
return $result;
}
// Test array
$num1 = array(4, 5, 4, 5, 2, 2, 3, 3, 2, 4, 4);
// Print the element with odd occurrences
print_r(odd_occurrence($num1) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition (odd_occurrence):
- The function "odd_occurrence()" takes an array '$arr' as input.
- It initializes a variable '$result' to store the result.
- Inside the function:
- It traverses the array using a foreach loop.
- For each element in the array, it performs a bitwise XOR operation (^) between the current element and the result.
- Bitwise XOR operation returns a bit that is set in either of the operands but not both. This helps find elements with odd occurrences efficiently.
- Function Call & Testing:
- The odd_occurrence function is tested with a test array '$num1'.
- The "print_r()" function prints the element with odd occurrences.
Sample Output:
2
Flowchart:
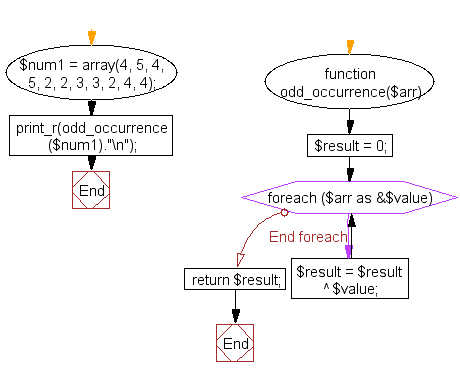
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to find the length of the last word in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics