PHP Challenges: Check whether a given positive integer is a power of three
Write a PHP program to check whether a given positive integer is a power of three.
Input : 9
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to check if a number is a power of three
function is_Power_of_three($n)
{
// Assign $n to a variable $x for manipulation
$x = $n;
// Loop until $x is divisible by 3
while ($x % 3 == 0) {
// Divide $x by 3
$x /= 3;
}
// Check if $x is equal to 1 after the loop
if ($x == 1) {
// If true, $n is a power of 3
return "$n is power of 3";
} else {
// If false, $n is not a power of 3
return "$n is not power of 3";
}
}
// Testing the function with different inputs
print_r(is_Power_of_three(9)."\n");
print_r(is_Power_of_three(81)."\n");
Explanation:
Here is a brief explanation of the above PHP code:
- The code defines a function named 'is_Power_of_three' that takes a single parameter '$n', which is the number to be checked for being a power of three.
- Inside the function 'is_Power_of_three':
- It assigns the value of '$n' to a variable '$x'.
- It enters a while loop that continues as long as '$x' is divisible by 3. Inside the loop, it divides '$x' by 3 in each iteration.
- After the loop, it checks if '$x' is equal to 1. If it is, then '$n' is a power of 3, so it returns a string indicating that. Otherwise, it returns a string indicating that '$n' is not a power of 3.
- Finally, the function is tested with three different values (9, 81, and 21) using the 'print_r' function to display the results of each test.
Sample Output:
9 is power of 3 81 is power of 3 21 is not power of 3
Flowchart:
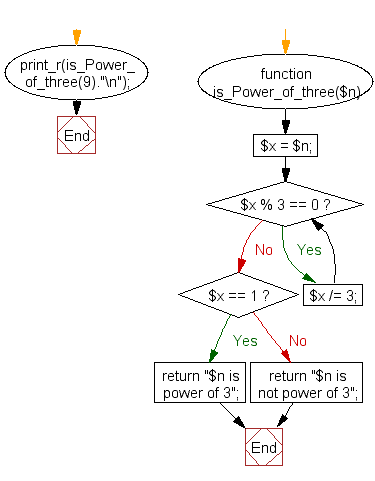
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check whether a given positive integer is a power of two.
Next: Write a PHP program to check whether a given positive integer is a power of four.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics