PHP Challenges: Compute the sum of the two reversed numbers and display the sum in reversed form
Write a PHP program to compute the sum of the two reversed numbers and display the sum in reversed form.
Input : 13, 14
Note : The result will not be unique for every number for example 31 is a reversed form of several numbers of 13, 130, 1300 etc. Therefore all the leading zeros will be omitted.
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to find the reverse sum of two numbers
function reverse_sum($n1, $n2)
{
// Return the sum of the reverse of both numbers
return reverse_integer($n1) + reverse_integer($n2);
}
// Function to reverse an integer
function reverse_integer($n)
{
// Initialize variable to store the reversed integer
$reverse = 0;
// Loop until the input number becomes 0
while ($n > 0)
{
// Multiply the current reversed number by 10 and add the last digit of the input number
$reverse = $reverse * 10;
$reverse = $reverse + $n % 10;
// Remove the last digit from the input number
$n = (int)($n / 10);
}
// Return the reversed integer
return $reverse;
}
// Test the reverse_sum function with different inputs and print the results
print_r(reverse_sum(13, 14) . "\n");
print_r(reverse_sum(130, 1) . "\n");
print_r(reverse_sum(305, 794) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "is_geometric()" which checks if an array forms a geometric sequence. It takes an array '$arr' as input.
- Inside the function:
- It first checks if the array size is 1 or less. If so, it returns 'True' as any sequence with 1 or fewer elements is considered geometric.
- It calculates the ratio between the second and first elements of the array.
- It iterates through the array elements starting from the second element.
- Within each iteration, it checks if the ratio of the current element to the previous element is equal to the initial ratio calculated.
- If any ratio is not equal to the initial ratio, it returns "Not a geometric sequence".
- If all ratios are equal, it returns "Geometric sequence".
- Function Call & Testing:
- Two arrays '$my_arr1' and '$my_arr2' are defined with different sets of numbers.
- The "is_geometric()" function is called twice with these arrays as input.
- Finally, "print_r()" function displays whether each input array forms a geometric sequence.
Sample Output:
72 32 1000
Flowchart:
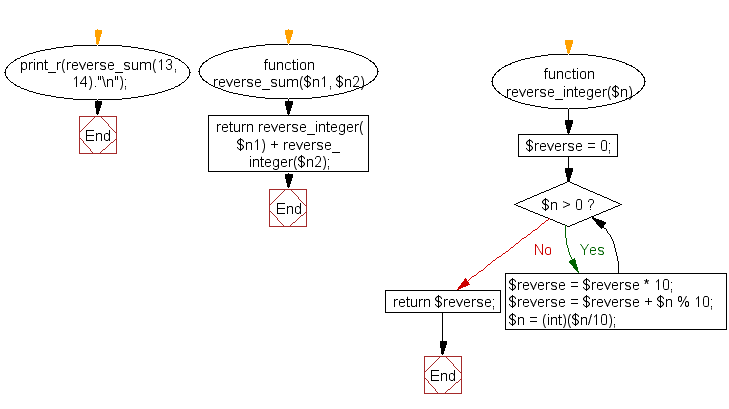
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a sequence of numbers is a geometric progression or not.
Next: Write a PHP program where you take any positive integer n, if n is even, divide it by 2 to get n / 2. If n is odd, multiply it by 3 and add 1 to obtain 3n + 1. Repeat the process until you reach 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.