PHP Challenges: Reverse the bits of an integer (32 bits unsigned)
Write a PHP program to reverse the bits of an integer (32 bits unsigned).
Input : 1234
For example, 1234 represented in binary as 10011010010 and returns 1260388352 which represents in binary as 1001011001000000000000000000000.
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to reverse the bits of an integer
function reverse_integer($n)
{
// Initialize a variable to store the reversed integer
$result = 0;
// Loop through 32 bits (assuming integers are represented by 32 bits)
for ($i = 0; $i < 32; $i++)
{
// Shift the result to the left by 1 bit
$result <<= 1;
// OR operation to set the least significant bit of result with the least significant bit of n
$result |= ($n & 1);
// Shift n to the right by 1 bit
$n >>= 1;
}
// Return the reversed integer
return $result;
}
// Test the reverse_integer function with input 1234 and print the result
print_r(reverse_integer(1234) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "reverse_integer()" which reverses the bits of an integer. It takes a single parameter '$n', which is the integer whose bits are to be reversed.
- Inside the function:
- It initializes a variable '$result' to store the reversed integer.
- It enters a "for" loop that iterates through 32 times, assuming integers are represented by 32 bits.
- Within each iteration of the loop:
- It shifts the bits of '$result' to the left by 1 position ($result <<= 1).
- It performs a bitwise OR operation to set the least significant bit of '$result' with the least significant bit of '$n' ($result |= ($n & 1)).
- It shifts the bits of '$n' to the right by 1 position ($n >>= 1).
- After the loop, it returns the reversed integer stored in '$result'.
- Function Call & Testing:
- The "reverse_integer()" function is called with the input integer 1234.
- Finally the "print_r()" function displays the reversed integer.
Sample Output:
1260388352
Flowchart:
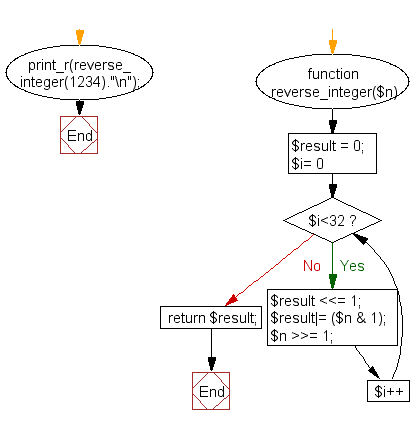
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to reverse the digits of an integer.
Next: Write a PHP program to check a sequence of numbers is an arithmetic progression or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.