PHP Challenges: Reverse the digits of an integer
Write a PHP program to reverse the digits of an integer.
Explanation :
Sample Solution :
PHP Code :
<?php
// Function to reverse an integer
function reverse_integer($n)
{
// Initialize variable to store the reversed integer
$reverse = 0;
// Loop until the input number becomes 0
while ($n > 0)
{
// Multiply the current reversed number by 10 and add the last digit of the input number
$reverse = $reverse * 10;
$reverse = $reverse + $n % 10;
// Remove the last digit from the input number
$n = (int)($n / 10);
}
// Return the reversed integer
return $reverse;
}
// Test the reverse_integer function with different inputs and print the results
print_r(reverse_integer(1234) . "\n");
print_r(reverse_integer(23) . "\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- Function definition:
- The code defines a function named "reverse_integer()" which reverses an integer. It takes a single parameter '$n', which is the integer to be reversed.
- Inside the function:
- It initializes a variable '$reverse' to store the reversed integer.
- It enters a "while" loop that continues until the input number '$n' becomes 0.
- Within each iteration of the loop:
- It multiplies the current reversed number '$reverse' by 10 and adds the last digit of the input number ($n % 10).
- It removes the last digit from the input number by integer division ($n / 10).
- After the loop, it returns the reversed integer stored in '$reverse'.
- Function Call & Testing:
- The "reverse_integer()" function is called twice with different input integers (1234 and 23).
- The results of the function calls are printed using "print_r()", which displays the reversed integers.
Sample Output:
4321 32
Flowchart:
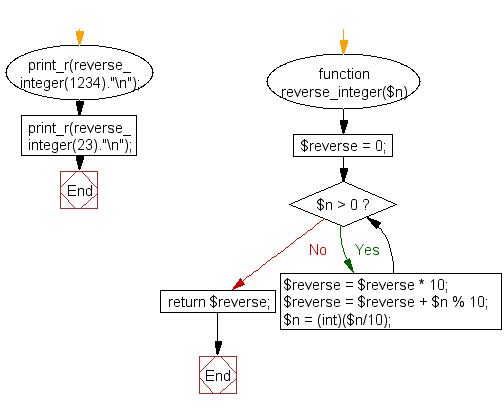
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to add the digits of a positive integer repeatedly until the result has a single digit.
Next: Write a PHP program to reverse the bits of an integer (32 bits unsigned).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics