PHP Challenges: Check whether a given positive integer is a power of two
Write a PHP program to check whether a given positive integer is a power of two.
Input : 4
Visualization of powers of two from 1 to 1024:
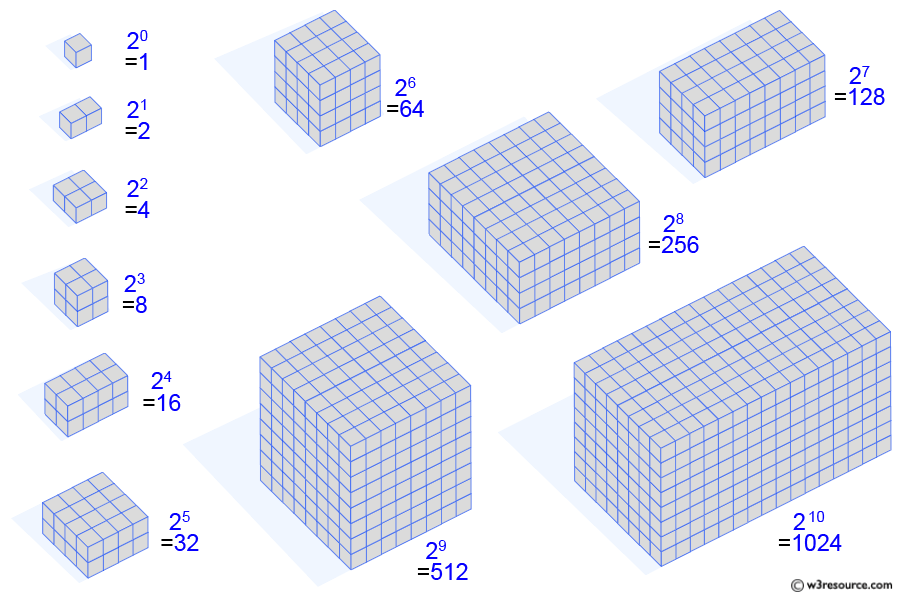
Sample Solution :
PHP Code :
<?php
// Function to check if a number is a power of two
function is_Power_of_two($n)
{
// Check if the bitwise AND of $n and $n - 1 equals 0
if(($n & ($n - 1)) == 0)
{
// If true, $n is a power of 2
return "$n is power of 2";
}
else
{
// If false, $n is not a power of 2
return "$n is not power of 2";
}
}
// Testing the function with different inputs
print_r(is_Power_of_two(4)."\n");
print_r(is_Power_of_two(36)."\n");
print_r(is_Power_of_two(16)."\n");
?>
Explanation:
Here is a brief explanation of the above PHP code:
- The code defines a function named 'is_Power_of_two' that takes a single parameter '$n', which is the number to be checked.
- Inside the function, it checks if '$n' is a power of two using bitwise operations. Specifically, it checks if the result of the expression '$n & ($n - 1)' equals zero. This expression is true only when '$n' is a power of two.
- If the condition is true, the function returns a string indicating that '$n' is a power of 2. If false, it returns a string indicating that '$n' is not a power of 2.
- Finally, the function is tested with three different values (4, 36, and 16) using the 'print_r' function to display the results of each test.
Sample Output:
4 is power of 2 36 is not power of 2 16 is power of 2
Flowchart:
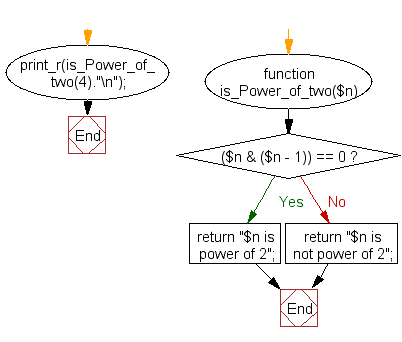
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP Challenges Exercises Home.
Next: Write a PHP program to check whether a given positive integer is a power of three.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics