PHP Exercises: Compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum
PHP Basic Algorithm: Exercise-99 with Solution
Write a PHP program to compute the sum of the two given arrays of integers, length 3 and find the array which has the largest sum.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes two arrays as parameters
function test($nums1, $nums2)
{
// Compare the sum of the first three elements of $nums1 with the sum of the first three elements of $nums2
// If the sum of $nums1 is greater or equal, return $nums1; otherwise, return $nums2
return $nums1[0] + $nums1[1] + $nums1[2] >= $nums2[0] + $nums2[1] + $nums2[2] ? $nums1 : $nums2;
}
// Call the 'test' function with arrays [10, 20, -30] and [10, 20, 30] and store the result in $result
$result = test([10, 20, -30], [10, 20, 30]);
// Print the new array (either $nums1 or $nums2) with maximum values using 'implode' and 'echo'
echo "New array with maximum values: " . implode(',', $result);
?>
Sample Output:
New array with maximum values: 10,20,30
Flowchart:
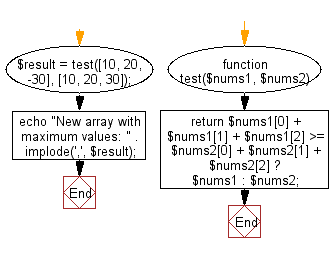
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a given array of integers, length 3 and create a new array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
Next: Write a PHP program to create an array taking two middle elements from a given array of integers of length even.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics