PHP Exercises: Check if a given array of integers and length 2, contains 15 or 20
95. Array Contains 15 or 20 Check
Write a PHP program to check if a given array of integers and length 2, contains 15 or 20.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Return true if the first element is equal to 15 or 20, or if the second element is equal to 15 or 20
return $nums[0] == 15 || $nums[0] == 20 || $nums[1] == 15 || $nums[1] == 20;
}
// Check and display the result of calling 'test' with the array [12, 20]
var_dump(test([12, 20]));
// Check and display the result of calling 'test' with the array [14, 15]
var_dump(test([14, 15]));
// Check and display the result of calling 'test' with the array [11, 21]
var_dump(test([11, 21]));
?>
Explanation:
- Function Definition:
- A function named test is defined, which takes one parameter:
- $nums: an array of numbers.
- Return Condition:
- The function checks whether:
- The first element of the array ($nums[0]) is equal to 15 or 20.
- The second element of the array ($nums[1]) is equal to 15 or 20.
- If any of these conditions is true, the function returns true; otherwise, it returns false.
Output:
bool(true) bool(true) bool(false)
Flowchart:
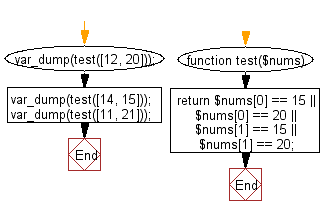
For more Practice: Solve these Related Problems:
- Write a PHP script to check if a two-element array contains either 15 or 20 and return a boolean accordingly.
- Write a PHP function to scan a small array and output true if either 15 or 20 exists within the elements.
- Write a PHP program to test the values of an array of length 2 and verify the presence of the numbers 15 or 20.
- Write a PHP script to use conditional statements to check for the existence of 15 or 20 in a given two-element array.
Go to:
PREV : New Array of First and Last Elements.
NEXT : Array Does Not Contain 15 or 20 Check.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.