PHP Exercises: Create a new array taking the first and last elements of a given array of integers and length 1 or more
Write a PHP program to create a new array taking the first and last elements of a given array of integers and length 1 or more.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes an array of numbers as a parameter
function test($nums)
{
// Return a new array containing the first and last elements of the input array
return [$nums[0], $nums[sizeof($nums) - 1]];
}
// Create an array $a with some numeric values
$a = [10, 20, -30, -40, 30];
// Print the original array as a string using 'implode' and 'echo'
echo "Original array: " . implode(',', $a) . "\n";
// Call the 'test' function with the array $a and store the result in $result
$result = test($a);
// Print the new array containing the first and last elements using 'implode' and 'echo'
echo "New array: " . implode(',', $result);
?>
Explanation:
- Function Definition:
- A function named test is defined that takes one parameter:
- $nums: an array of numbers.
- Returning Elements:
- The function returns a new array containing:
- The first element of the input array ($nums[0]).
- The last element of the input array ($nums[sizeof($nums) - 1]).
- Array Initialization:
- An array $a is created with the following numeric values:
- $a = [10, 20, -30, -40, 30];
- Display Original Array:
- The contents of the original array $a are printed as a string using implode:
- Function Call:
- The test function is called with the array $a, and the result is stored in the variable $result.
- Display New Array:
- The new array, which contains the first and last elements of the original array, is printed using implode.
Output:
Original array: 10,20,-30,-40,30 New array: 10,30
Flowchart:
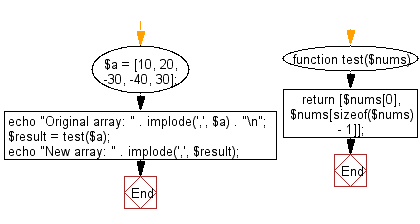
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to find out the maximum element between the first or last element in a given array of integers ( length 4), replace all elements with maximum element.
Next: Write a PHP program to check if a given array of integers and length 2, contains 15 or 20.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics