PHP Exercises: Create a new array containing the middle elements from the two given arrays of integers, each length 5
PHP Basic Algorithm: Exercise-93 with Solution
Write a PHP program to create a new array containing the middle elements from the two given arrays of integers, each length 5.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that takes two arrays as parameters
function test($nums1, $nums2)
{
// Return a new array containing the third element from both input arrays
return [$nums1[2], $nums2[2]];
}
// Create two arrays $nums1 and $nums2 with numeric values
$nums1 = [10, 20, -30, -40, 30];
$nums2 = [10, 20, 30, 40, 30];
// Print the original contents of array $nums1 as a string using 'implode' and 'echo'
echo "Original array1: " . implode(',', $nums1) . "\n";
// Print the original contents of array $nums2 as a string using 'implode' and 'echo'
echo "Original array2: " . implode(',', $nums1) . "\n";
// Call the 'test' function with arrays $nums1 and $nums2 and store the result in $result
$result = test($nums1, $nums2);
// Print the new array containing the third element from both input arrays using 'implode' and 'echo'
echo "New array: " . implode(',', $result);
?>
Explanation:
- Function Definition:
- A function named test is defined, which takes two parameters:
- $nums1: the first input array.
- $nums2: the second input array.
- Returning Elements:
- The function returns a new array that contains:
- The third element from $nums1 ($nums1[2]).
- The third element from $nums2 ($nums2[2]).
- Array Initialization:
- Two arrays are created:
- $nums1 = [10, 20, -30, -40, 30]
- $nums2 = [10, 20, 30, 40, 30]
- Display Original Arrays:
- The contents of $nums1 are printed using implode to format it as a string:
- The contents of $nums2 are intended to be printed, but there is a mistake in the code. It incorrectly prints $nums1 again instead of $nums2.
- Function Call:
- The test function is called with the two arrays, and the result is stored in the variable $result.
- Display New Array:
- The new array, which contains the third elements from both input arrays, is printed using implode:
Output:
Original array1: 10,20,-30,-40,30 Original array2: 10,20,-30,-40,30 New array: -30,30
Flowchart:
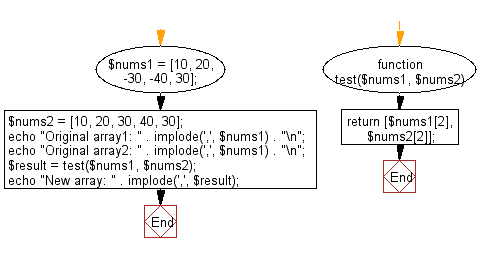
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to find out the maximum element between the first or last element in a given array of integers ( length 4), replace all elements with maximum element.
Next: Write a PHP program to create a new array taking the first and last elements of a given array of integers and length 1 or more.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-93.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics