PHP Exercises: Create a new string with the last char added at the front and back of a given string of length 1 or more
9. Add Last Character at Front and Back
Write a PHP program to create a new string with the last char added at the front and back of a given string of length 1 or more.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $str
function test($str)
{
// Use the substr function to get the last character of $str
$s = substr($str, strlen($str) - 1);
// Concatenate the last character, $str, and the last character again, then return the result
return $s . $str . $s;
}
// Call the test function with argument "Red" and echo the result
echo test("Red") . "\n";
// Call the test function with argument "Green" and echo the result
echo test("Green") . "\n";
// Call the test function with argument "1" and echo the result
echo test("1") . "\n";
?>
Explanation:
- Function Definition:
- The function test takes one parameter, $str.
- Extracting the Last Character:
- Uses substr($str, strlen($str) - 1) to get the last character of $str and assigns it to $s.
- Concatenation:
- Concatenates $s (last character), $str (the original string), and $s (last character again) to create a new string with the last character at both the beginning and end.
- Returns this concatenated result.
- Function Calls and Output:
- First Call: test("Red")
- Last character is "d", so the result is "dRedd".
- Second Call: test("Green")
- Last character is "n", so the result is "nGreenn".
- Third Call: test("1")
- Last character is "1" (the only character), so the result is "111".
Output:
dRedd nGreenn 111
Visual Presentation:
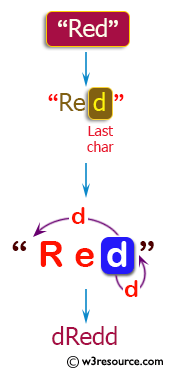
Flowchart:
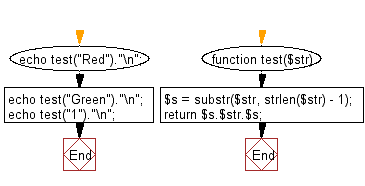
For more Practice: Solve these Related Problems:
- Write a PHP script to take the last character of a string and append and prepend it to the original string, using string indexing.
- Write a PHP function to surround a string with its final character and handle strings of length one by simple repetition.
- Write a PHP program to concatenate the last character to both ends of a string and test with varying string lengths.
- Write a PHP script to manipulate a given string so its first and last characters become its prefix and suffix, respectively.
Go to:
PREV : Four Copies of First 2 Characters.
NEXT : Check Multiple of 3 or 7.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.