PHP Exercises: Check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element
Write a PHP program to check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that checks if the first element of either array is equal
// to the first element of the other array, or if the last element of either array is equal
// to the last element of the other array.
function test($a1, $a2)
{
// Check if the first element of either array is equal to the first element of the other array,
// or if the last element of either array is equal to the last element of the other array.
return $a1[0] == $a2[0] || $a1[sizeof($a1) - 1] == $a2[sizeof($a2) - 1];
}
// Test the 'test' function with different arrays, then display the results
var_dump(test([10, 20, 40, 50], [10, 20, 40, 50]));
var_dump(test([10, 20, 40, 10], [10, 20, 40, 5]));
var_dump(test([12, 24, 35, 55], [1, 20, 40, 5]));
?>
Explanation:
- Function Definition:
- The function test is defined with two parameters:
- $a1: the first array.
- $a2: the second array.
- Condition Check:
- The function checks two conditions using logical OR (||):
- First Element Equality: It checks if the first element of $a1 ($a1[0]) is equal to the first element of $a2 ($a2[0]).
- Last Element Equality: It checks if the last element of $a1 ($a1[sizeof($a1) - 1]) is equal to the last element of $a2 ($a2[sizeof($a2) - 1]).
- Return Statement:
- The function returns true if either condition is satisfied; otherwise, it returns false.
Output:
bool(true) bool(true) bool(false)
Flowchart:
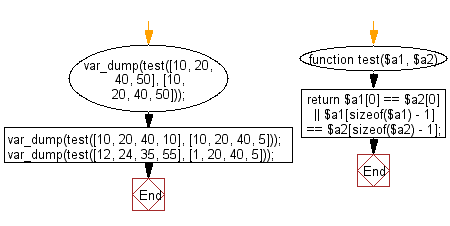
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array.
Next: Write a PHP program to compute the sum of the elements of an given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics