PHP Exercises: Create a new string from a specified string
PHP Basic Algorithm: Exercise-83 with Solution
Write a PHP program to create a new string from a given string. If the two characters of the given string from its beginning and end are same return the given string without the first two characters otherwise return the original string.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that checks if the first two characters of a string are equal to the last two characters
function test($s1)
{
// Check if the length of $s1 is greater than 1 and if the first two characters are equal to the last two characters
if (strlen($s1) > 1 && substr($s1, 0, 2) == substr($s1, strlen($s1) - 2))
{
// If the conditions are met, return a substring starting from the third character
return substr($s1, 2);
}
// If the conditions are not met
else
{
// Return the original string
return $s1;
}
}
// Test the 'test' function with different strings, then display the results
echo test("abcab") . "\n";
echo test("Python") . "\n";
?>
Explanation:
- Function Definition:
- The function test is defined with one parameter:
- $s1: the input string to be processed.
- Condition Check:
- The function checks two conditions:
- Condition 1: Whether the length of $s1 is greater than 1.
- Condition 2: Whether the first two characters of $s1 are equal to the last two characters. This is done using:
- substr($s1, 0, 2) to get the first two characters.
- substr($s1, strlen($s1) - 2) to get the last two characters.
- Return Value:
- If Both Conditions Are True:
- The function returns a substring starting from the third character onward using:
- return substr($s1, 2);
- If Any Condition Is False:
- The function returns the original string unchanged:
- return $s1;
Output:
cab Python
Flowchart:
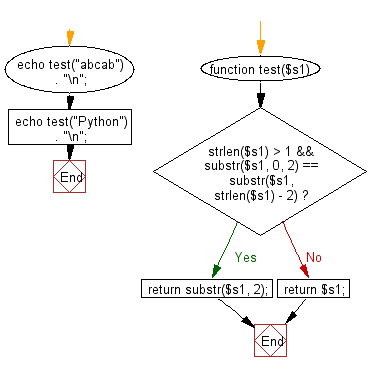
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string using 3 copies of the first 2 characters of a given string. If the length of the given string is less than 2 use the whole string.
Next: Write a PHP program to create a new string from a given string without the first and last character if the first or last characters are 'a' otherwise return the original given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/basic-algorithm/php-basic-algorithm-exercise-83.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics