PHP Exercises: Concat two given strings. If the given strings have different length remove the characters from the longer string
81. Concat with Removal for Different Lengths
Write a PHP program to concat two given strings. If the given strings have different length remove the characters from the longer string.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that concatenates two strings in a specific way
// The resulting string will have the length of the longer input string
function test($s1, $s2)
{
// Check if the length of $s1 is less than the length of $s2
if (strlen($s1) < strlen($s2))
{
// Concatenate $s1 with the end portion of $s2 to make their lengths equal
return $s1 . substr($s2, strlen($s2) - strlen($s1));
}
// Check if the length of $s1 is greater than the length of $s2
else if (strlen($s1) > strlen($s2))
{
// Concatenate the end portion of $s1 with $s2 to make their lengths equal
return substr($s1, strlen($s1) - strlen($s2)) . $s2;
}
// If lengths are equal, simply concatenate the two strings
else
{
return $s1 . $s2;
}
}
// Test the 'test' function with different pairs of strings, then display the results
echo test("abc", "abcd") . "\n";
echo test("Python", "Python") . "\n";
echo test("JS", "Python") . "\n";
?>
Explanation:
- Function Definition:
- The function test is defined with two parameters:
- $s1: the first input string.
- $s2: the second input string.
- Length Comparison:
- The function checks the lengths of the two strings to determine how to concatenate them.
- Concatenation Logic:
- Case 1: If the length of $s1 is less than the length of $s2:
- The function concatenates $s1 with the end portion of $s2 to make their lengths equal:
- return $s1 . substr($s2, strlen($s2) - strlen($s1));
- Case 2: If the length of $s1 is greater than the length of $s2:
- The function concatenates the end portion of $s1 with $s2 to make their lengths equal:
- return substr($s1, strlen($s1) - strlen($s2)) . $s2;
- Case 3: If the lengths of $s1 and $s2 are equal:
- The function simply concatenates the two strings:
- return $s1 . $s2;
Output:
abcbcd PythonPython JSon
Flowchart:
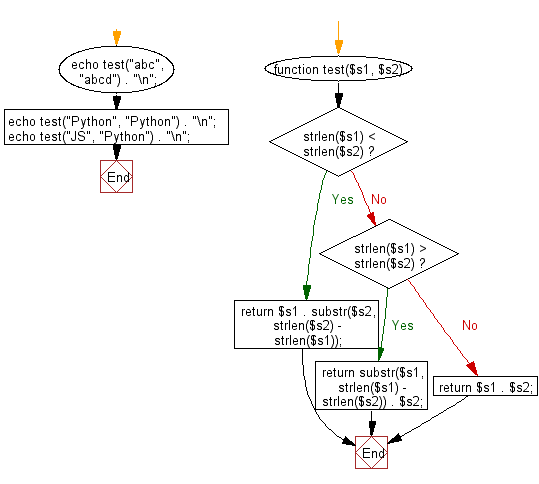
For more Practice: Solve these Related Problems:
- Write a PHP script to concatenate two strings after removing excess characters from the longer one until both have equal lengths.
- Write a PHP function to compare two input strings and trim the longer string so that both have matching lengths before joining them.
- Write a PHP program to remove characters from the end of the longer string to match the shorter string's length and then concatenate the two.
- Write a PHP script to implement logic that balances two strings by truncating the longer one and then merging them.
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to check whether the first two characters and last two characters of a given string are same.
Next: Write a PHP program to create a new string using 3 copies of the first 2 characters of a given string. If the length of the given string is less than 2 use the whole string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.