PHP Exercises: Check if a given string begins with 'abc' or 'xyz'
79. Check String Starts with 'abc' or 'xyz'
Write a PHP program to check if a given string begins with 'abc' or 'xyz'. If the string begins with 'abc' or 'xyz' return 'abc' or 'xyz' otherwise return the empty string.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that checks the prefix of a string
function test($s1)
{
// Check if the first three characters of $s1 are "abc"
if (substr($s1, 0, 3) == "abc")
{
// If true, return "abc"
return "abc";
}
// Check if the first three characters of $s1 are "xyz"
else if (substr($s1, 0, 3) == "xyz")
{
// If true, return "xyz"
return "xyz";
}
// If the conditions are not met, return an empty string
else
{
return "";
}
}
// Test the 'test' function with different strings, then display the results using echo
echo test("abc")."\n";
echo test("abcdef")."\n";
echo test("C")."\n";
echo test("xyz")."\n";
echo test("xyzsder")."\n";
?>
Explanation:
- The function test is defined with one parameter:
- $s1: the input string to check.
- The function checks if the first three characters of $s1 are "abc":
- if (substr($s1, 0, 3) == "abc")
- If true:
- It returns the string "abc".
- If the first condition is false, it checks if the first three characters of $s1 are "xyz":
- else if (substr($s1, 0, 3) == "xyz")
- If true:
- It returns the string "xyz".
- If neither condition is met:
- It returns an empty string "".
Output:
abc abc xyz xyz
Flowchart:
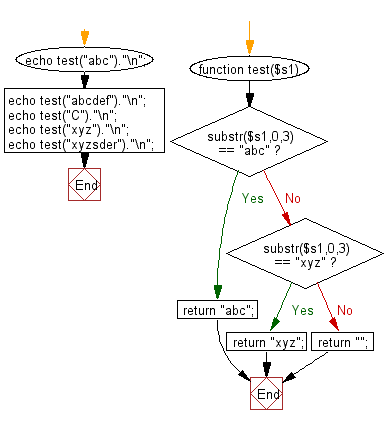
For more Practice: Solve these Related Problems:
- Write a PHP script to determine if a string begins with "abc" or "xyz" and return the matching prefix, or an empty string otherwise.
- Write a PHP function to scan the start of a string for either "abc" or "xyz" and output the detected substring.
- Write a PHP program to check a string's prefix and return it if it equals one of the two target values using substring comparison.
- Write a PHP script to use regex to match the beginning of the string with either "abc" or "xyz" and output the match accordingly.
Go to:
PREV : Swap Last Two Characters in String.
NEXT : First Two and Last Two Characters Equality.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.