PHP Exercises: Create a new string from a given string after swapping last two characters
78. Swap Last Two Characters in String
Write a PHP program to create a new string from a given string after swapping last two characters.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that manipulates a string based on its length
function test($s1)
{
// Check if the length of $s1 is greater than 1
if (strlen($s1) > 1)
{
// If true, return a modified string:
// Concatenate the substring of $s1 from the beginning to the second-to-last character,
// then append the last character, and finally, append the second-to-last character
return substr($s1, 0, strlen($s1) - 2) . substr($s1, strlen($s1) - 1, 1) . substr($s1, strlen($s1) - 2, 1);
}
else
{
// If false (length is less than or equal to 1), return $s1 as is
return $s1;
}
}
// Test the 'test' function with different strings, then display the results using echo
echo test("Hello")."\n";
echo test("Python")."\n";
echo test("PHP")."\n";
echo test("JS")."\n";
echo test("C")."\n";
?>
Explanation:
- Function Definition:
- The function test is defined with a single parameter:
- $s1: the input string to manipulate.
- Length Check:
- The function checks if the length of $s1 is greater than 1.
- strlen($s1) > 1
- String Manipulation (if condition is true):
- If the length of $s1 is greater than 1:
- Extracts and concatenates parts of the string as follows:
- First Part: substr($s1, 0, strlen($s1) - 2)
- This gets the substring from the start of $s1 up to the second-to-last character.
- Last Character: substr($s1, strlen($s1) - 1, 1)
- This retrieves the last character of the string.
- Second-to-Last Character: substr($s1, strlen($s1) - 2, 1)
- This retrieves the second-to-last character of the string.
- The modified string is formed by concatenating these three parts.
- Return Original String (if condition is false):
- If the length of $s1 is less than or equal to 1:
- The function returns $s1 unchanged.
Output:
Helol Pythno PPH SJ C
Flowchart:
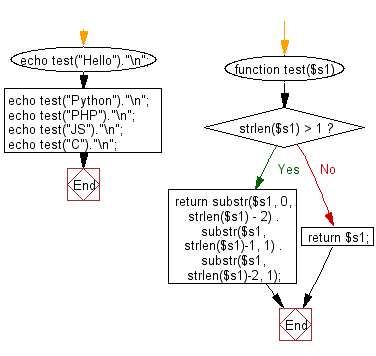
For more Practice: Solve these Related Problems:
- Write a PHP script to swap the last two characters of a string and return the modified string.
- Write a PHP function to identify the final two letters and exchange their positions, leaving shorter strings unchanged.
- Write a PHP program to manipulate an input string by interchanging its last two characters using substr functions.
- Write a PHP script to check string length and, if adequate, reverse the order of the final two characters.
Go to:
PREV : Concat Lowercase Strings and Omit Doubles.
NEXT : Check String Starts with 'abc' or 'xyz'.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.