PHP Exercises: Concat two given strings
77. Concat Lowercase Strings and Omit Doubles
Write a PHP program to concat two given strings (lowercase). If there are any double character in new string then omit one character.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that concatenates two strings based on certain conditions
function test($s1, $s2)
{
// Check if the length of $s1 is less than 1
if (strlen($s1) < 1)
{
// If true, return $s2
return $s2;
}
// Check if the length of $s2 is less than 1
if (strlen($s2) < 1)
{
// If true, return $s1
return $s1;
}
// Check if the last character of $s1 is not equal to the first character of $s2
if (substr($s1, strlen($s1) - 1, 1) <> substr($s2, 0, 1))
{
// If true, concatenate $s1 and $s2
return $s1 . $s2;
}
else
{
// If false, concatenate $s1 and the substring of $s2 from the second character
return $s1 . substr($s2, 1, strlen($s1) - 1);
}
}
// Test the 'test' function with different strings, then display the results using echo
echo test("abc", "cat")."\n";
echo test("Python", "PHP")."\n";
echo test("php", "php")."\n";
?>
Explanation:
- Function Definition:
- The test function is defined with two parameters:
- $s1: the first input string.
- $s2: the second input string.
- Condition 1: Check if $s1 is Empty:
- If $s1 has less than 1 character (i.e., it is empty):
- Return $s2.
- Condition 2: Check if $s2 is Empty:
- If $s2 has less than 1 character (i.e., it is empty):
- Return $s1.
- Condition 3: Check the Last Character of $s1 and the First Character of $s2:
- If the last character of $s1 is not equal to the first character of $s2:
- Concatenate $s1 and $s2 directly.
- Otherwise (if they are equal):
- Concatenate $s1 with $s2, excluding the first character of $s2 (to avoid duplication).
Output:
abcat PythonPHP phphp
Flowchart:
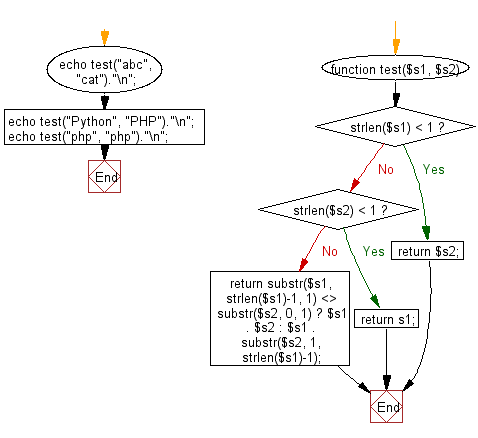
For more Practice: Solve these Related Problems:
- Write a PHP script to merge two lowercase strings and remove any consecutive duplicate characters from the result.
- Write a PHP function to combine two input strings, then scan for and eliminate any double-character occurrences.
- Write a PHP program to concatenate two strings and apply a filter to reduce repeated characters in overlapping areas.
- Write a PHP script to process lowercase inputs, join them, and employ regex to collapse adjacent duplicates into a single instance.
Go to:
PREV : Concat First from One and Last from Another.
NEXT : Swap Last Two Characters in String.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.