PHP Exercises: Create a new string taking 3 characters from the middle of a given string at least 3
74. Middle 3 Characters from a String
Write a PHP program to create a new string taking 3 characters from the middle of a given string at least 3.
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that extracts a substring of length 3 from the middle of the input string
// If the length of the string is odd, the middle is calculated as (length - 1) / 2 - 1
// If the length is even, the middle is calculated as length / 2 - 1
function test($s1)
{
// Use substr to extract a substring of length 3 from the middle of the string
// If the length is odd, calculate the middle as (length - 1) / 2 - 1
// If the length is even, calculate the middle as length / 2 - 1
return substr($s1, (strlen($s1) - 1) / 2 - 1, 3);
}
// Test the 'test' function with different strings, then display the results using echo
echo test("Hello")."\n";
echo test("Python")."\n";
echo test("abc")."\n";
?>
Explanation:
- Function Definition:
- A function named test is defined with a single parameter:
- $s1: the input string.
- Substring Extraction:
- The function calculates the starting index for extracting a 3-character substring from the middle of the input string:
- If the string length is odd, (strlen($s1) - 1) / 2 - 1 gives the starting position of the middle three characters.
- If the string length is even, the same formula approximates the middle.
- substr($s1, (strlen($s1) - 1) / 2 - 1, 3) extracts three characters starting from the calculated middle index.
Output:
ell yth abc
Flowchart:
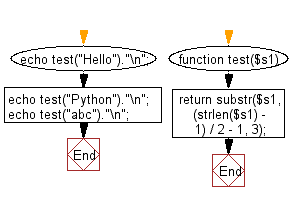
For more Practice: Solve these Related Problems:
- Write a PHP script to extract three characters from the middle of a string, ensuring the string's length is at least 3.
- Write a PHP function to calculate the middle index of a string and slice out a three-character segment centered on it.
- Write a PHP program to validate string length and output a fixed-length substring taken from its middle.
- Write a PHP script to handle odd-length strings by approximating the middle and then extracting three letters.
Go to:
PREV : Substring of Length 2 at Given Index.
NEXT : New String of Length 2 with Padding.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.