PHP Exercises: Check if a given string ends with "on"
71. Check if String Ends with "on"
Write a PHP program to check if a given string ends with "on".
Sample Solution:
PHP Code :
<?php
// Define a function named 'test' that extracts the last two characters from the input string
function test($s1)
{
// Use substr to obtain the last two characters of the input string
return substr($s1, strlen($s1)-2, 2);
}
// Test the 'test' function with different strings and display the results using var_dump
var_dump(test("Hello"));
var_dump(test("Python"));
var_dump(test("on"));
var_dump(test("o"));
?>
Explanation:
- Function Definition:
- A function named test is defined with one parameter, $s1, which represents the input string.
- Extracting the Last Two Characters:
- The function uses substr($s1, strlen($s1) - 2, 2):
- strlen($s1) - 2 calculates the starting position, which is two characters from the end of the string.
- 2 specifies that two characters should be extracted from this position.
- Return Value:
- The function returns the last two characters of $s1. If $s1 has less than two characters, it returns the whole string.
Output:
string(2) "lo" string(2) "on" string(2) "on" string(1) "o"
Flowchart:
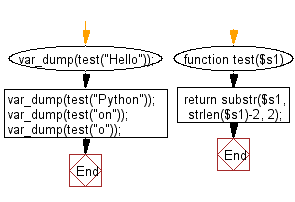
For more Practice: Solve these Related Problems:
- Write a PHP script to verify whether an input string terminates with the substring "on" using substr comparisons.
- Write a PHP function to extract the ending letters of a string and check if they match "on".
- Write a PHP program to compare the last two characters of a string with the target substring "on" and return a boolean.
- Write a PHP script to use regex to determine if the ending pattern of a string is "on".
Go to:
PREV : Middle Two Characters from Even-Length String.
NEXT : Concat First and Last n Characters.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.