PHP Exercises: Exchange the first and last characters in a given string and return the new string
Write a PHP program to exchange the first and last characters in a given string and return the new string.
Sample Solution:
PHP Code :
<?php
// Define a function named "test" that takes a parameter $str
function test($str)
{
// Use a ternary operator to check if the length of $str is greater than 1
// If true, concatenate the last character with the substring from index 1 to length-2
// and then concatenate the first character at the end
// If false, return $str as it is
return strlen($str) > 1 ? substr($str, strlen($str) - 1) . substr($str, 1, strlen($str) - 2) . substr($str, 0, 1) : $str;
}
// Call the test function with argument "abcd" and echo the result
echo test("abcd") . "\n";
// Call the test function with argument "a" and echo the result
echo test("a") . "\n";
// Call the test function with argument "xy" and echo the result
echo test("xy") . "\n";
?>
Explanation:
- Function Definition:
- The function test takes one parameter, $str.
- Conditional Check:
- It uses a ternary operator to check if the length of $str is greater than 1.
- If true:
- Constructs a new string by:
- Taking the last character of $str using substr($str, strlen($str) - 1).
- Appending the substring from index 1 to the second-last character with substr($str, 1, strlen($str) - 2).
- Adding the first character at the end using substr($str, 0, 1).
- This effectively swaps the first and last characters of $str.
- If false:
- Returns $str as is (for strings of length 1 or less).
- Function Calls and Output:
- First Call: test("abcd")
- Swaps the first ("a") and last ("d") characters, resulting in "dbca".
- Second Call: test("a")
- Returns "a" since the length is 1.
- Third Call: test("xy")
- Swaps "x" and "y", resulting in "yx".
Output:
dbca a yx
Visual Presentation:
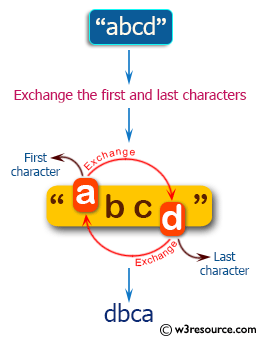
Flowchart:
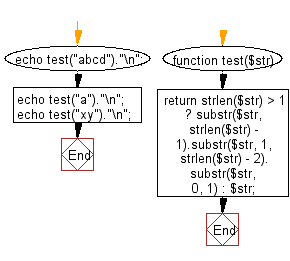
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to remove the character in a given position of a given string. The given position will be in the range 0..string length -1 inclusive.
Next: Write a PHP program to create a new string which is 4 copies of the 2 front characters of a given string. If the given string length is less than 2 return the original string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics